I’m currently working on an AutoFixture Pluralsight course and it got me thinking about using it to generate anonymous test data when writing automated UI tests.
The basic premise is that in addition to using AutoFixture to generate unit test data, it can also be used to populate UI elements where the specific data values are unimportant.
If you are unfamiliar with BDDfy and Seleno, they are part of the TestStack project.
BDDfy allows tests to be written and to produce business readable documentation. Seleno allows the automation of web browsers using Selenium and strongly-typed page object models.
Example Scenario
Imagine that we have an (ASP.NET MVC) web site that allows the addition of members of the royal family.
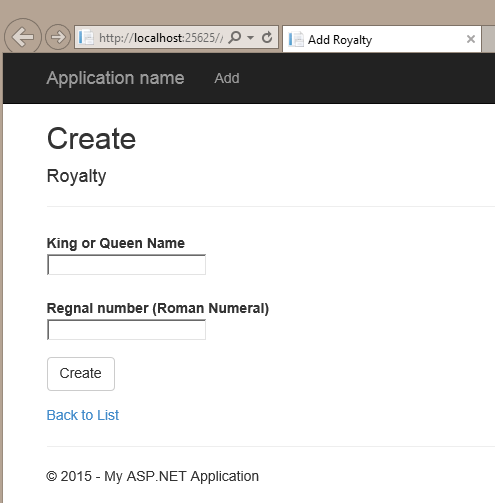
If we wanted to test different king/queen names (but didn’t care about the Regnal number) we could start off by defining some strongly typed (Seleno) page object models:
public class HomePage : Page<AddRoyaltyModel>
{
public CreatedPage CreateRoyalty(AddRoyaltyModel royal)
{
Input.Model(royal);
return Navigate.To<CreatedPage>(By.Id("Create"));
}
}
public class CreatedPage : Page
{
}
(all the examples in this post are fairly quick-and-dirty to demonstrate the AutoFixture involvement)
Next we can write some example in BDDfy (using xUnit.net as the test framework):
public class AddRoyaltyTests
{
private HomePage _home;
private CreatedPage _confirmationPage;
public string Name { get; set; }
public string Number { get; set; }
[Fact]
public void ShouldAddRoyaltiesWithDifferentNames()
{
this.Given(x => GivenIAmStartingANewRoyalAddition())
.And("And I have entered <name> as the royalty name")
.And("And I have entered <number> as the regnal number")
.When(x => WhenIChooseToAddTheNewRoyal())
.Then(x => ThenIShouldSeeAConfirmationOfTheNewRoyalHavingBeenAdded())
.WithExamples(new ExampleTable("name", "number")
{
{"Richard", "I"},
{"Henry", "I"},
{"Elizabeth", "I"}
})
.BDDfy();
}
private void GivenIAmStartingANewRoyalAddition()
{
_home = Host.Instance.NavigateToInitialPage<HomeController, HomePage>(x => x.Index());
}
private void WhenIChooseToAddTheNewRoyal()
{
var royal = new AddRoyaltyModel
{
Name = this.Name,
RegnalRomanNumeral = this.Number
};
_confirmationPage = _home.CreateRoyalty(royal);
}
private void ThenIShouldSeeAConfirmationOfTheNewRoyalHavingBeenAdded()
{
Assert.Equal("Created ok", _confirmationPage.Title);
}
}
Notice in the preceding code that even though we don’t care about the Regnal number we are still supplying it in the examples. We could just set it manually to a hardcoded value, and in this simple example that might be ok, but if we had a form with many fields then this will introduce extra work and may make the test less “refactor-safe”.
The following code shows the removal of the Regnal name/number:
public class AddRoyaltyTestsUsingAutoFixture
{
private HomePage _home;
private CreatedPage _confirmationPage;
public string Name { get; set; }
[Fact]
public void ShouldAddRoyaltiesWithDifferentNames()
{
this.Given(x => GivenIAmStartingANewRoyalAddition())
.And("And I have entered <name> as the royalty name")
.When(x => WhenIChooseToAddTheNewRoyal())
.Then(x => ThenIShouldSeeAConfirmationOfTheNewRoyalHavingBeenAdded())
.WithExamples(new ExampleTable("name")
{
"Richard",
"Henry",
"Elizabeth"
})
.BDDfy();
}
private void GivenIAmStartingANewRoyalAddition()
{
_home = Host.Instance.NavigateToInitialPage<HomeController, HomePage>(x => x.Index());
}
private void WhenIChooseToAddTheNewRoyal()
{
var fixture = new Fixture();
// Use AutoFixture to create anonymous data for all properties except
// name which is set to the BDDfy example value
var royal = fixture.Build<AddRoyaltyModel>()
.With(x => x.Name, this.Name)
.Create();
_confirmationPage = _home.CreateRoyalty(royal);
}
private void ThenIShouldSeeAConfirmationOfTheNewRoyalHavingBeenAdded()
{
Assert.Equal("Created ok", _confirmationPage.Title);
}
}
In this version, AutoFixture’s Build method is being used to automatically generate test data for all the fields, except the royal name which is set to the name(s) specified in the BDDfy examples.
Running this test results in the following automation:
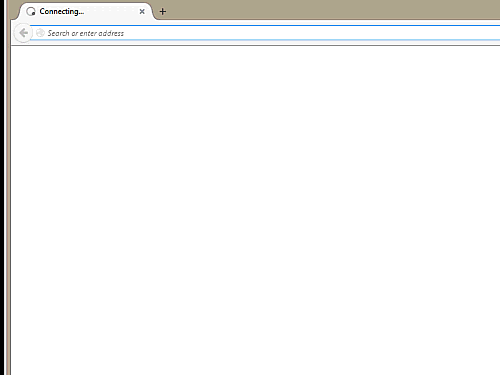
And produces the following HTML BDDfy report:
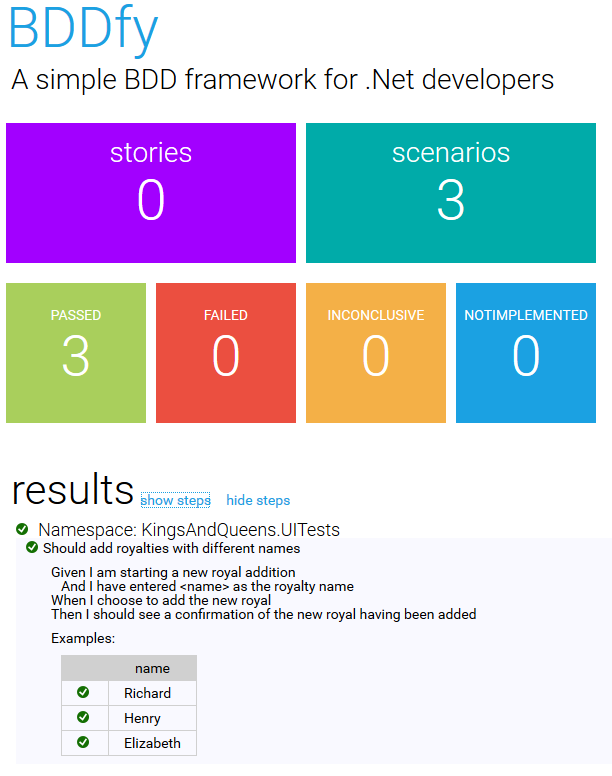
If you want to fill in the gaps in your C# knowledge be sure to check out my C# Tips and Traps training course from Pluralsight – get started with a free trial.


SHARE: