Universal Windows apps promise to enable the sharing of app code between Windows 8.1 Store apps and Windows Phone 8.1 apps.
In this demo, we’ll create a simple app and see how much work we can share between the two platforms. The sample code and design is probably not how we’d choose to implement a final solution but it will suffice for demo purposes.
Getting Started
Install Visual Studio 2013 Update 2.
Create a new project, head to the Visual C#, Store Apps, Universal Apps; choose the Blank App (Universal Apps) as the following screenshot shows. Name the app “Speechless”.
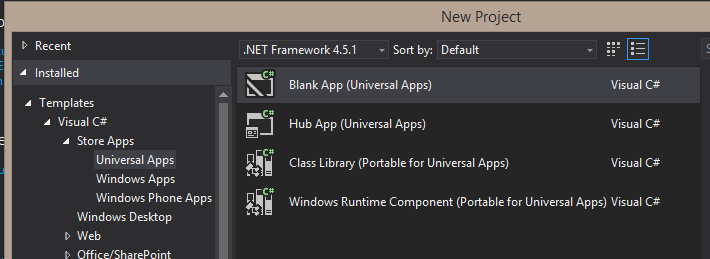
Once the solution is created, there will be 3 projects, one for Windows Store, one for Windows Phone, and a third project where we can put all the code we want to share between the two apps.
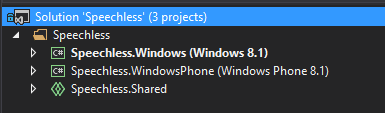
Creating a Shared Class
First off let’s create a class that we can share between apps:
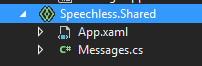
using System.Collections.Generic;
namespace Speechless
{
static class Messages
{
public static IEnumerable<string> Greetings
{
get
{
yield return "Hello";
yield return "Yo!";
yield return "What's up?";
yield return "Good day sir!";
}
}
}
}
This just gives a few greetings that the user can choose from. Because this class was added in the Speechless.Shared project, it can be used from both apps.
So we could just go and create some XAML in each app and use this class, but let’s see if we can actually share XAML between apps to further reduce effort.
Creating a shared User Control
In the Speechless.Shared project, add a new item of type User Control called “ChooseGreatingControl.xaml”.
Add some XAML:
<UserControl
x:Class="Speechless.ChooseGreatingControl"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:Speechless"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
d:DesignHeight="300"
d:DesignWidth="400"
Width="300">
<Grid>
<StackPanel>
<ComboBox Name="GreetingChoice" ></ComboBox>
<Button Name="SayIt" Click="SayIt_OnClick">Speak</Button>
</StackPanel>
</Grid>
</UserControl>
This gives the user a ComboBox to choose which greeting to speak.
In the code behind:
using System;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
namespace Speechless
{
public sealed partial class ChooseGreatingControl : UserControl
{
public ChooseGreatingControl()
{
this.InitializeComponent();
GreetingChoice.ItemsSource = Messages.Greetings;
GreetingChoice.SelectedIndex = 0;
}
private async void SayIt_OnClick(object sender, RoutedEventArgs e)
{
}
}
}
Here we’re just wiring up the ComboBox items to those in the Messages class and setting the default selected item to the first one.
Next let’s try to add some code to do some text to speech:
private async void SayIt_OnClick(object sender, RoutedEventArgs e)
{
var sayWhat = GreetingChoice.SelectedValue as string;
var synth = new Windows.Media.SpeechSynthesis.SpeechSynthesizer();
Windows.Media.SpeechSynthesis.SpeechSynthesisStream stream = await synth.SynthesizeTextToStreamAsync(sayWhat);
var mediaElement = new MediaElement();
mediaElement.SetSource(stream, stream.ContentType);
mediaElement.Play();
}
So now we have some UI XAML and some code, let’s see how easy it is to share this user control between the two apps.
Using the Same User Control from Both Windows Store and Windows Phone Apps
In the MainPage.xaml of the Windows Store app project, let’s add an instance of the user control:
<Page
x:Class="Speechless.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:Speechless"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<local:ChooseGreatingControl></local:ChooseGreatingControl>
</Grid>
</Page>
Let’s go and do the same for the Windows Phone app:
<Page
x:Class="Speechless.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:Speechless"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<Grid>
<local:ChooseGreatingControl></local:ChooseGreatingControl>
</Grid>
</Page>
Running the Apps
So lets try running now, first the Windows Store app (which is selected as the default start-up app), so F5…
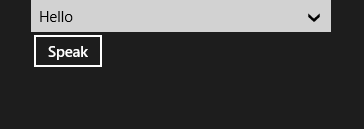
Clicking the Speak button works, and speaks outputs the selected greeting.
So let’s try the Windows Phone app, select it as the start-up app and hit F5…
System.UnauthorizedAccessException
This is because we need to add the Microphone capability to the Windows Phone app, once this is done the app runs and works as expected:
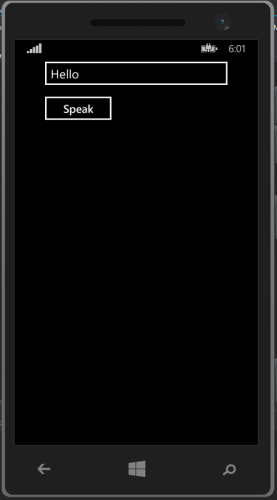
Conclusions?
This is pretty cool. Having written an app in the past that targets both Windows Store and Windows Phone this shared project makes things SO much easier – no linked file frustrations.
The next app I start writing (whether Store or Phone) I’ll use this Universal template just so if I want to port it to the other platform it will be ready to go.
It’s going to be interesting mixing this approach with Portable Class Libraries as well. Also a framework such as MVVMLight.
If there is code in the Shared project that only applies to either Store or Phone, we can use the predefined directives:
#if WINDOWS_APP
// Store only app specific code here
#else
// Phone only app specific code here
#endif
Whilst I don’t know what the plans are regarding Xbox One, it would be cool to have an Xbox One app store and we could just add an “Xbox One App” to our Universal Apps solution, build, and have the same app with most of the code and UI shared between Windows Store, Windows Phone, and Xbox One.
Exciting times!
SHARE: