We want our test code to be as high quality as possible, this means smaller amounts of code duplication, reasonably easy to find where things are in Visual Studio, etc.
One possible organization structure is to think in terms of the individual features in the application. The approach you take will probably depend on the complexity and size of the test suite, system under test, etc. Because BDDfy is "just code" we can use all the normal techniques of composition and inheritance that we'd use in our production code to get to the right level of code reuse and organization for the application we're testing.
Organisation by Feature
Organising by feature enables a reasonable amount of code reuse between test scenarios and it also helps to think user- or business-first rather than code/implementation first.
So for example, if we’re using BDDfy to test a banking application we might have the following features:
- Login
- Logout
- Move Money
- Pay Bills
- View Transactions
- etc
Each of these features contains a number of scenarios, for example Login would probably contain scenarios for successful login, bad password, locked out account, 2 factor login, etc.
In Visual Studio we could create folders in the test project to represent and organize these features as the following screenshot illustrates.
So a (cut down) Login BDDfy story class could look like the following code:
namespace Tests.Login
{
[TestFixture]
[Story(AsA="As a Customer",
IWant = "I want to login",
SoThat = "So that I can manage my accounts and money")]
public class CustomerLogin
{
[Test]
public void LoginSuccess()
{
this.Given(x => GivenIAmOnTheLoginScreen())
.And(x => x.AndIHaveEnteredMyUsername())
.And(x => AndIHaveEnteredMyPassword())
.When( x=> WhenIChooseLogin())
.Then(x => ThenIShouldBeLoggedIn())
.BDDfy<CustomerLogin>();
}
public void GivenIAmOnTheLoginScreen()
{
}
public void AndIHaveEnteredMyUsername()
{
}
public void AndIHaveEnteredMyPassword()
{
}
public void WhenIChooseLogin()
{
}
public void ThenIShouldBeLoggedIn()
{
}
}
}
Now for arguments sake, say we have a Navigation story class that represents how the user should be able to move around the applications features.
We could reuse the individual given/when/then methods in CustomerLogin but we don’t want our navigations scenarios to be bloated, we want them to represent the essence of the scenario with the right level of detail.
So the first thing we could do is to create a “step aggregation” method in CustomerLogin as follows:
public void GivenIHaveLoggedIn()
{
GivenIAmOnTheLoginScreen();
AndIHaveEnteredMyUsername();
AndIHaveEnteredMyPassword();
WhenIChooseLogin();
}
This method simply re-uses the existing steps but aggregates them into a method we can call from the navigation tests:
using NUnit.Framework;
using TestStack.BDDfy;
using TestStack.BDDfy.Core;
using Tests.Login;
using TestStack.BDDfy.Scanners.StepScanners.Fluent;
namespace Tests
{
[TestFixture]
[Story(AsA="As a Customer",
IWant = "I want to navigate around the site",
SoThat = "So that I can get to the features I want to use")]
public class Navigation
{
[Test]
public void NavigateToMoveMoney()
{
var custLogin = new CustomerLogin();
this.Given(x => custLogin.GivenIHaveLoggedIn())
.When(x => WhenChooseGotoMoveMoney())
.Then(x => ThenIShouldBeTakenToTheMainMoveMoneyScreen())
.BDDfy<Navigation>();
}
private void ThenIShouldBeTakenToTheMainMoveMoneyScreen()
{
}
private void WhenChooseGotoMoveMoney()
{
}
}
}
So here were are making use of this aggregate method from the CustomerLogin feature class.
If we don’t need to represent the fact that the customer is logged-in in the report and all the tests in the class assume a logged-in user, we could use some (e.g. NUnit) test setup code that logs the user in but doesn’t get reported.
The HTML output of this looks as follows:
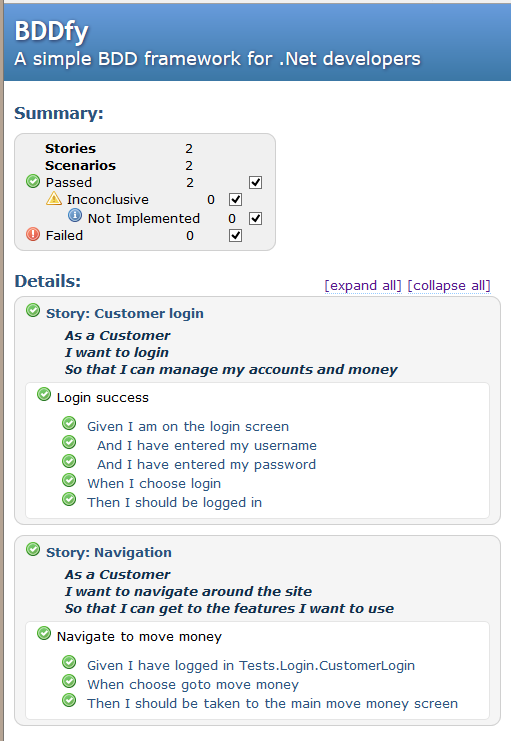
While in these examples we have a single story class for the feature (e.g. CustomerLogin) once we start adding scenarios (and steps) this single story class might become too bloated. If this is deemed a problem then we can break it out into sub features/stories or if it’s applicable we could use inheritance to hold common given/when/then steps. The individual story classes relating to the customer login feature would all inherit this base class. We probably however, do not want multiple levels of nested inheritance in our stories as this may make maintenance and discoverability harder.
To see more of what BDDfy can do check out my Building the Right Thing in .NET with TestStack Pluralsight course, head on over to the documentation, or check it out on GitHub.
SHARE: