With Windows Store apps there are challenges getting traditional mocking frameworks such as Rhino and Moq working due to limited reflection support in the platform (presumably for security reasons).
I wrote a mocking solution when Windows Phone 7 first came out, it can also be used for Windows Store apps. I’ve updated the NuGet descriptions etc. to reflect this.
How To Do TDD with Mocking in Windows Store Apps
Create a new (C#/XAML) Windows Store app project in Visual Studio called “MyAwesomeApp”.
Create your test project “MyAwesomeApp.Tests” and reference your main app.
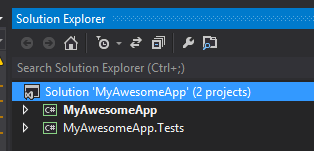
In the main app project, install the MoqaLate NuGet package. When the package is installed you will have a new folder in the main app solution called “MoqaLateCommandLine”:
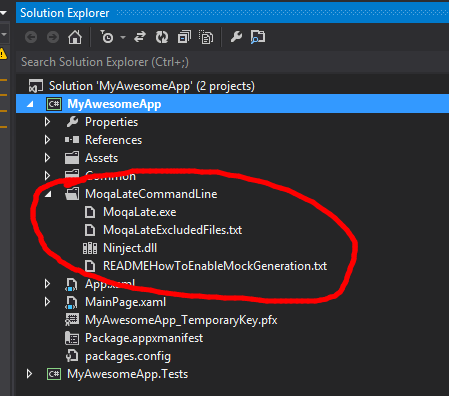
(Inside this folder is a readmexxx.txt file with some additional info)
MoqaLate works as a post-build step in your main app project. It looks through all the code files for any interfaces you’ve defined. For each of these interfaces it generates a class to act as a fake (mock), and copies these fakes to a directory in your test project.
Right-click on MyAwesomeApp and choose Properties. Goto the Build Events and add the following as a Post Build event: $(ProjectDir)\MoqaLateCommandLine\MoqaLate.exe "$(SolutionDir)$(ProjectName)" "$(SolutionDir)MyAwesomeApp.Tests\Fakes"
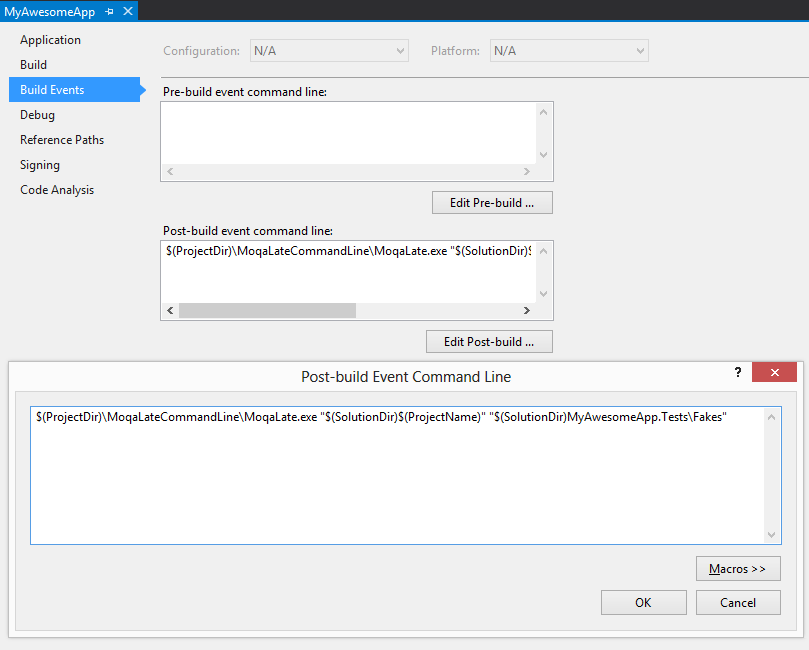
Now every time MyAwesomeApp is built, fakes will be generated in the test project in a Fakes directory. To test this, create the following interface in the MyAwesomeApp project:
namespace MyAwesomeApp
{
public interface IFoo
{
bool Bar();
int SuperFoo(string s);
}
}
Now build the solution. There should be no errors.
Expand the MyAwesomeApp.Tests project, and choose the show all files icon, you’ll now see a fake called FooMoqaLate.cs :
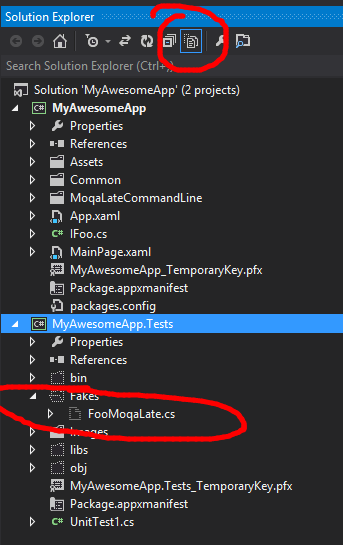
Right-click the fakes folder and choose Include In Project. You now have a fake implementation of IFoo (called FooMoqaLate) that you can use in your tests as follows.
In TDD fashion let’s create a test in the test project (I’m using xUnit.net but you can use any testing framework):
using MoqaLate.Autogenerated;
using Xunit;
namespace MyAwesomeApp.Tests
{
public class NeedFooTests
{
private const int Once = 1;
[Fact]
public void ShouldCallFooBarWhenPrinting()
{
var mockFoo = new FooMoqaLate();
var sut = new NeedFoo(mockFoo);
sut.Print();
Assert.True(mockFoo.BarWasCalled(Once));
}
}
}
The test checks that sut (System Under Test, i.e. the class NeedFoo) calls the Bar method on it’s IFoo dependency. To make this code compile we need to create the following class in the main project:
namespace MyAwesomeApp
{
public class NeedFoo
{
private readonly IFoo _foo;
public NeedFoo(IFoo foo)
{
_foo = foo;
}
public void Print()
{
// something
}
}
}
If we run the test it fails, we next write some code to make the test pass, we change the Print method to:
public void Print()
{
_foo.Bar();
}
The test now passes.
MoqaLate also support the following methods on it’s fakes/mocks:
//mockFoo.BarSetReturnValue(false);
//var howManyTimeWasBarCalled = mockFoo.BarTimesCalled();
// for interface methods with parameters:
//Assert.True(mockFoo.SuperFooWasCalledWith("hello"));
The original article on MoqaLate is here.
(If you’re developing a Windows 8 app and you’d like to learn more about how to choose features for version one of your app, etc check out my Designing Windows 8 Apps Pluralsight course.)
SHARE: