To compliment the release of my Testing .NET Code with xUnit.net 2 Pluralsight course, I’ve updated my original xUnit.net cheat sheet.
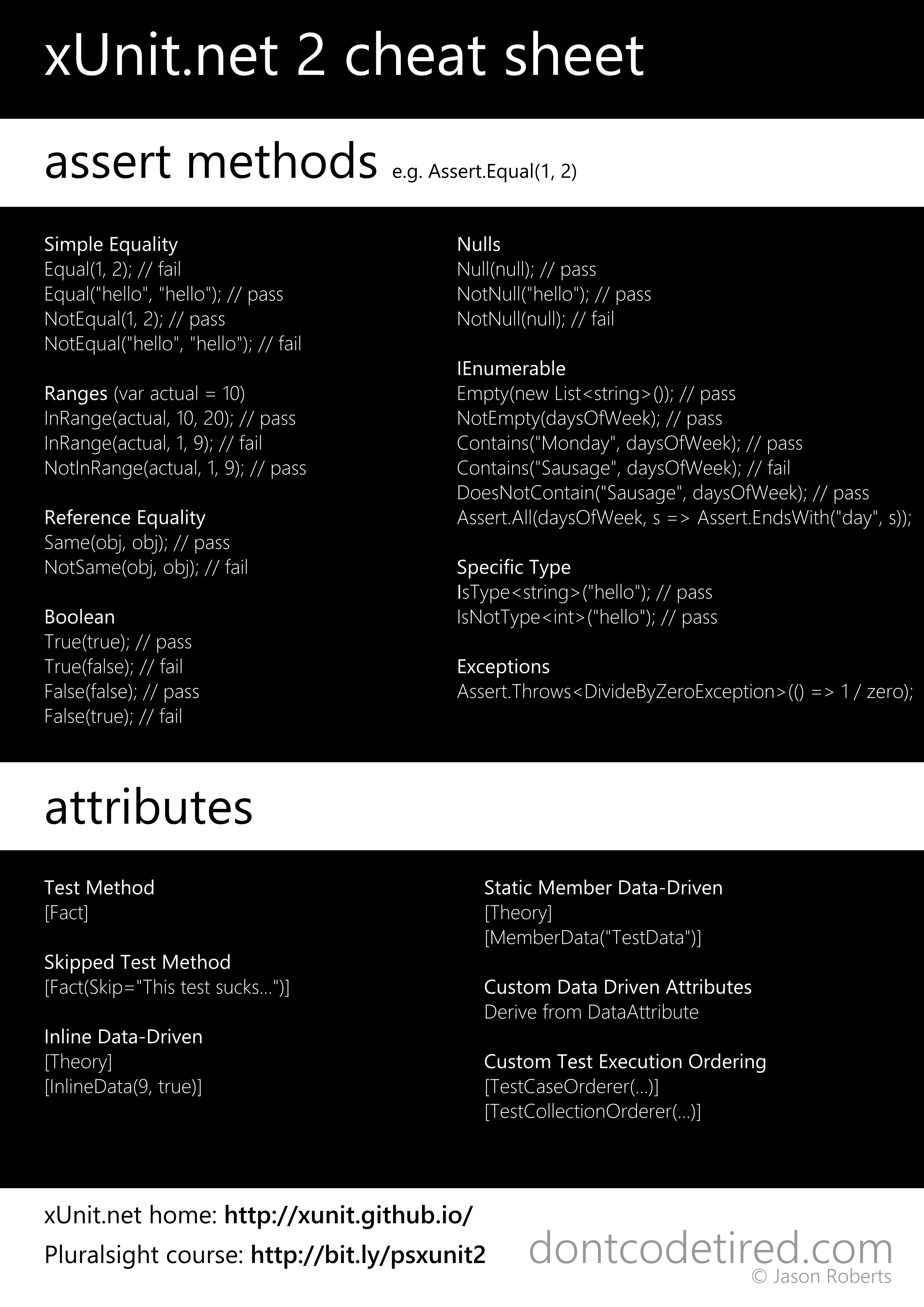
(To download just right-click, save-as)
To learn xUnit.net check out my xUnit.net Pluralsight training course.
You can start watching with a Pluralsight free trial.


SHARE:
xUnit.net allows the creation of data-driven tests. These kind of tests get their test data from outside the test method code via parameters added to the test method signature.
Say we had to test a Calculator class and check it’s add method returned the correct results for six different test cases. Without data-driven tests we’d either have to write six separates tests (with almost identical code) or some loop inside our test method containing an assert.
Regular xUnit.net test methods are identified by applying the [Fact] attribute. Data-driven tests instead use the [Theory] attribute.
To get data-driven features and the [Theory] attribute, install the xUnit.net Extensions NuGet package in addition to the standard xUnit.net package.
Creating Inline Data-Driven Tests
The [InlineData] attribute allows us to specify test data that gets passed to the parameters of test method.
So for our Calculator add test we’d start by defining the test method:
More...
SHARE:
To help people get started with xUnit.net and as an accompaniment to my Pluralsight xUnit.net training course I thought I’d create a cheat sheet showing common assert methods and attributes. Hopefully will be of use :)
More...
SHARE:
My latest Pluralsight course on the xUnit.net testing framework has just been released.
Course Description
Learn the latest in unit testing technology for C#, VB.NET (and other .NET languages) created by the original inventor of NUnit.
xUnit.net is a free, extensible, open source framework designed for programmers that aligns more closely with the .NET platform.
You can check it out now on Pluralsight.com.
You can start watching with a Pluralsight free trial.


SHARE:
My newest Pluralsight course has just been published.
We shouldn't live in fear of our code
Long-term customer satisfaction, agility, and developer happiness are crucial. A quality suite of automated tests helps achieve this. This practical course covers how and what to test at the unit, integration, and functional UI levels; and how to bring them all together with TeamCity continuous integration build server.
The course helps to keep your software soft with the right automated tests at the right level.
You can start watching with a Pluralsight free trial.


SHARE:
Just knocked up this quick and dirty video explaining how to set up MoqaLate in a Windows Store app solution.
You can find out more about MoqaLate on the MoqaLate project home page.
SHARE:
With Windows Store apps there are challenges getting traditional mocking frameworks such as Rhino and Moq working due to limited reflection support in the platform (presumably for security reasons).
I wrote a mocking solution when Windows Phone 7 first came out, it can also be used for Windows Store apps. I’ve updated the NuGet descriptions etc. to reflect this.
How To Do TDD with Mocking in Windows Store Apps
Create a new (C#/XAML) Windows Store app project in Visual Studio called “MyAwesomeApp”.
Create your test project “MyAwesomeApp.Tests” and reference your main app.
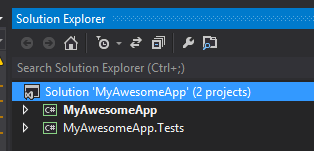
In the main app project, install the MoqaLate NuGet package. When the package is installed you will have a new folder in the main app solution called “MoqaLateCommandLine”:
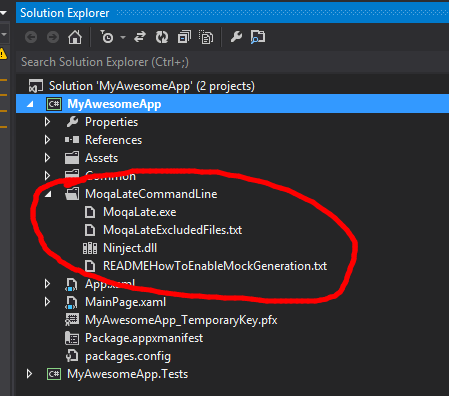
(Inside this folder is a readmexxx.txt file with some additional info)
More...
SHARE:
Introducing MoqaLate
Whilst I love developing apps for Windows Phone 7, the testing aspect is hard! I'm a TDD-er by default and it's such a pain to have to hand roll my own mock objects.
So I created MoqaLate.
It's an alpha version but is usable now.
Not sure framework is the right term but it's something that generates mocks from your interfaces.
Add to existing project from NuGet:
PM> Install-Package MoqaLate
Read more about the project.
Download an example solution.
Read (currently very basic!) documentation.
View on NuGet.org
Awesome overview diagram :)
SHARE:
One thing that can quickly become messy when writing unit tests is the creation of test objects. If the test object is a simple int, string, etc then it's not as much of a problem as when you a list or object graph you need to create.
Even using object initializers you can end up taking a lot of lines of indented code, and gets in the way of the tests.
One solution is to use a 'builder' class which will construct the object for you.
For example, rather than lots of initializer code you could write:
_sampleData = new HistoryBuilder()
.WithTimer(false, 1, 1, new DateTime(2000, 1, 1))
.WithTimer(false, 1, 1, new DateTime(2000, 1, 1))
.WithTimer(true, 1, 1, new DateTime(2000, 1, 1))
.Build()
You can multiple overloads of WithTimer (for example one which create adds a default Timer).
Implementation of HistoryBuilder:
public class HistoryBuilder
{
private readonly History _history;
public HistoryBuilder()
{
_history = new History();
}
public HistoryBuilder WithTimer()
{
_history.Timers.Add(new Timer());
return this;
}
public HistoryBuilder WithTimer(bool completed, int internalInteruptions, int externalInteruptions,
DateTime time)
{
_history.Timers.Add(new Timer
{
Completed = completed,
InternalInteruptionsCount = internalInteruptions,
ExternalInteruptionsCount = externalInteruptions,
StartedTime = time
});
return this;
}
public History Build()
{
return _history;
}
}
If you want to fill in the gaps in your C# knowledge be sure to check out my C# Tips and Traps training course from Pluralsight – get started with a free trial.


SHARE: