AutoFixture is a library that you can use alongside your testing framework to reduce the amount of boilerplate test code you need to write and thus improve your productivity.
At its core, AutoFixture helps you setup your tests by generating anonymous test data for you. This anonymous test data can be used to fulfil non-important boilerplate test data; this is test data that is required for the test to execute but whose value is unimportant.
Take the follow abbreviated test:
[Fact]
public void ManualCreation()
{
// arrange
Customer customer = new Customer()
{
CustomerName = "Amrit"
};
Order order = new Order(customer)
{
Id = 42,
OrderDate = DateTime.Now,
Items =
{
new OrderItem
{
ProductName = "Rubber ducks",
Quantity = 2
}
}
};
// act and assert phases...
}
Suppose the previous test code was only creating an Order (with associated Customer) just to fulfil some dependency and the actual Customer and OrderItems did not matter. In this case we could use AutoFixture to generate them for us.
AutoFixture can be installed via NuGet and once installed allows a Fixture instance to be instantiated. This Fixture object can then be used to generate anonymous test data and greatly simplify the arrange phase, as the following test shows:
[Fact]
public void AutoCreation()
{
// arrange
var fixture = new Fixture();
Order order = fixture.Create<Order>();
// act and assert phases...
}
If we were to debug this test we’d see the following values:
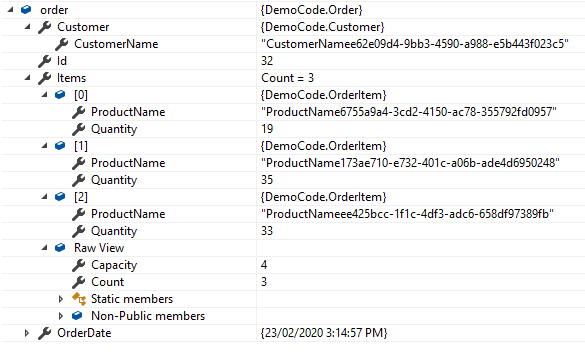
Notice in the preceding screenshot that AutoFixture has created the object graph for us, including the Customer and 3 OrderItem instances.
There’s a lot more to AutoFixture than just this simple example, for example you can combine with the AutoFixture.Xunit2 package to further reduce code:
[Theory, AutoData]
public void SubtractWhenZeroTest(int aPositiveNumber, Calculator sut)
{
// Act
sut.Subtract(aPositiveNumber);
// Assert
Assert.True(sut.Value < 0);
}
If you want to learn more about how AutoFixture can improve your productivity check out the docs or start watching for free my AutoFixture Pluralsight course with a free trial:


SHARE: