(This post refers to Azure Functions v2)
One way to test Azure Functions that use Event Grid triggers is to run the Function App locally and then get Azure in the cloud to invoke the function running on the local machine. As an example, suppose you want to use Event Grid to improve the reliability and responsiveness of Blob Storage processing. To do this the documentation suggests the use of ngrok. Now when a blob is added to a container in the cloud, the locally running function on the dev machine will be invoked via ngrok.
There is a somewhat simpler solution that allows you to invoke the Event Grid triggered function locally.
This approach bypasses Event Grid completely, so it is not a substitute for proper end-to-end testing, it’s more a development-time testing & debugging tool.
Manually Running Non HTTP-Triggered Azure Functions
You can manually trigger a non HTTP-triggered function (such as a timer triggered or Event Grid triggered function) via a special HTTP endpoint.
The endpoint is of the format: {host}/admin/functions/{function name}
For example, take the following function (which was also used in the post Improving Azure Functions Blob Trigger Performance and Reliability - Part 3: Using Event Grid to Respond to New Blobs):
public static class ProcessFoodBlobsEventGrid
{
private static readonly string[] _meats = { "steak", "chicken", "venison" };
[FunctionName("ProcessFoodBlobsEventGrid")]
public static void Run(
[EventGridTrigger]EventGridEvent blobCreatedEvent,
[Blob("{data.url}")] string foods, // assumes small blob size so using string not stream
[Blob("{data.url}.vegetarian")] out string vegetarian,
[Blob("{data.url}.nonvegetarian")] out string nonVegetarian,
ILogger log)
{
log.LogInformation("Processing a blob created event");
StorageBlobCreatedEventData createdEvent = ((JObject)blobCreatedEvent.Data).ToObject<StorageBlobCreatedEventData>();
log.LogInformation($"Blob: {createdEvent.Url}");
log.LogInformation($"Api operation: {createdEvent.Api}");
vegetarian = null;
nonVegetarian = null;
string[] foodLines = foods.Split(new[] { "\r\n", "\n" }, StringSplitOptions.RemoveEmptyEntries);
foreach (var food in foodLines)
{
var isMeat = _meats.Contains(food);
if (isMeat)
{
nonVegetarian += food + Environment.NewLine;
}
else
{
vegetarian += food + Environment.NewLine;
}
}
}
}
The preceding function when running locally in development would have the special URL: http://localhost:7071/admin/functions/ProcessFoodBlobsEventGrid
If you had a timer-triggered function called HerdCats that you wanted to manually invoke (so you didn’t have to wait for the next timed invocation) the special URL would be:http://localhost:7071/admin/functions/HerdCats
Note: when running locally in development you do not have to authenticate. If you wanted to manually invoke a deployed function in Azure, you need to provide an x-functions-key header that contains the function master key.
Manually Invoking an Event Grid Triggered Azure Function
When using the special URL to invoke a function, you can also provide data to be passed to the function. The type of data passed will depend on the trigger type of the function that you are invoking.
To provide data to the function, a JSON payload can be posted to the special URL. The data that is passed to the function is contained in a JSON property called “input”:
{
"input": "trigger data goes here"
}
If the Event Grid triggered function will be invoked by a new blob event, the contents of this input property must match the event schema for an Azure Blob Storage event.
An example of event JSON (taken from the Microsoft documentation):
[{
"topic": "/subscriptions/{subscription-id}/resourceGroups/Storage/providers/Microsoft.Storage/storageAccounts/xstoretestaccount",
"subject": "/blobServices/default/containers/testcontainer/blobs/testfile.txt",
"eventType": "Microsoft.Storage.BlobCreated",
"eventTime": "2017-06-26T18:41:00.9584103Z",
"id": "831e1650-001e-001b-66ab-eeb76e069631",
"data": {
"api": "PutBlockList",
"clientRequestId": "6d79dbfb-0e37-4fc4-981f-442c9ca65760",
"requestId": "831e1650-001e-001b-66ab-eeb76e000000",
"eTag": "0x8D4BCC2E4835CD0",
"contentType": "text/plain",
"contentLength": 524288,
"blobType": "BlockBlob",
"url": "https://example.blob.core.windows.net/testcontainer/testfile.txt",
"sequencer": "00000000000004420000000000028963",
"storageDiagnostics": {
"batchId": "b68529f3-68cd-4744-baa4-3c0498ec19f0"
}
},
"dataVersion": "",
"metadataVersion": "1"
}]
When testing the function outlined earlier, the first thing to do is ensure that there is a blob in the local blob container that will be read by the function by way of the blob input binding: [Blob("{data.url}")] string foods
.
For example, in the Storage Emulator a blob called in.txt can be uploaded to the food-in container.
Now the new blob event data JSON needs to be modified, specifically the data.url property needs to contain the URL to the local blob: http://127.0.0.1:10000/devstoreaccount1/food-in/in.txt
A modified version with updated data.url would be as follows:
[{
"topic": "/subscriptions/{subscription-id}/resourceGroups/Storage/providers/Microsoft.Storage/storageAccounts/xstoretestaccount",
"subject": "/blobServices/default/containers/testcontainer/blobs/testfile.txt",
"eventType": "Microsoft.Storage.BlobCreated",
"eventTime": "2017-06-26T18:41:00.9584103Z",
"id": "831e1650-001e-001b-66ab-eeb76e069631",
"data": {
"api": "PutBlockList",
"clientRequestId": "6d79dbfb-0e37-4fc4-981f-442c9ca65760",
"requestId": "831e1650-001e-001b-66ab-eeb76e000000",
"eTag": "0x8D4BCC2E4835CD0",
"contentType": "text/plain",
"contentLength": 524288,
"blobType": "BlockBlob",
"url": "http://127.0.0.1:10000/devstoreaccount1/food-in/in.txt",
"sequencer": "00000000000004420000000000028963",
"storageDiagnostics": {
"batchId": "b68529f3-68cd-4744-baa4-3c0498ec19f0"
}
},
"dataVersion": "",
"metadataVersion": "1"
}]
The next step is to remove the surrounding [], and replace the “ with ‘. Then paste the resulting JSON into the input property:
{
"input": "
{
'topic': '/subscriptions/{subscription-id}/resourceGroups/Storage/providers/Microsoft.Storage/storageAccounts/xstoretestaccount',
'subject': '/blobServices/default/containers/oc2d2817345i200097container/blobs/oc2d2817345i20002296blob',
'eventType': 'Microsoft.Storage.BlobCreated',
'eventTime': '2017-06-26T18:41:00.9584103Z',
'id': '831e1650-001e-001b-66ab-eeb76e069631',
'data': {
'api': 'PutBlockList',
'clientRequestId': '6d79dbfb-0e37-4fc4-981f-442c9ca65760',
'requestId': '831e1650-001e-001b-66ab-eeb76e000000',
'eTag': '0x8D4BCC2E4835CD0',
'contentType': 'application/octet-stream',
'contentLength': 524288,
'blobType': 'BlockBlob',
'url': 'http://127.0.0.1:10000/devstoreaccount1/food-in/in.txt',
'sequencer': '00000000000004420000000000028963',
'storageDiagnostics': {
'batchId': 'b68529f3-68cd-4744-baa4-3c0498ec19f0'
}
},
'dataVersion': '',
'metadataVersion': '1'
}
"
}
Now this JSON can be POSTed to the special URL: in the case of the example in this post the URL would be: http://localhost:7071/admin/functions/ProcessFoodBlobsEventGrid
The following screenshot shows posting using Postman:
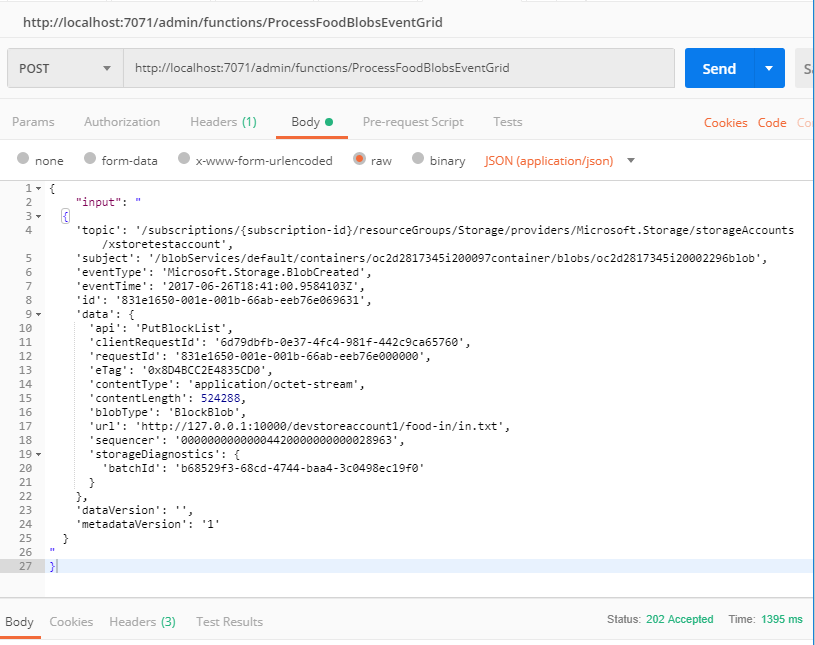
Posting will cause the Event Grid triggered function to be invoked and the JSON contained inside the input property will be passed to the trigger input EventGridEvent blobCreatedEvent object. The function will execute and read in the blob called “in.txt”.
If you want to fill in the gaps in your C# knowledge be sure to check out my C# Tips and Traps training course from Pluralsight – get started with a free trial.


SHARE: