This is the fourth part in a series of articles.
The Cosmos DB Azure Functions trigger can be used in conjunction with the Azure SignalR Service to create real-time notifications of changes made to data in Cosmos DB, all in a serverless way.
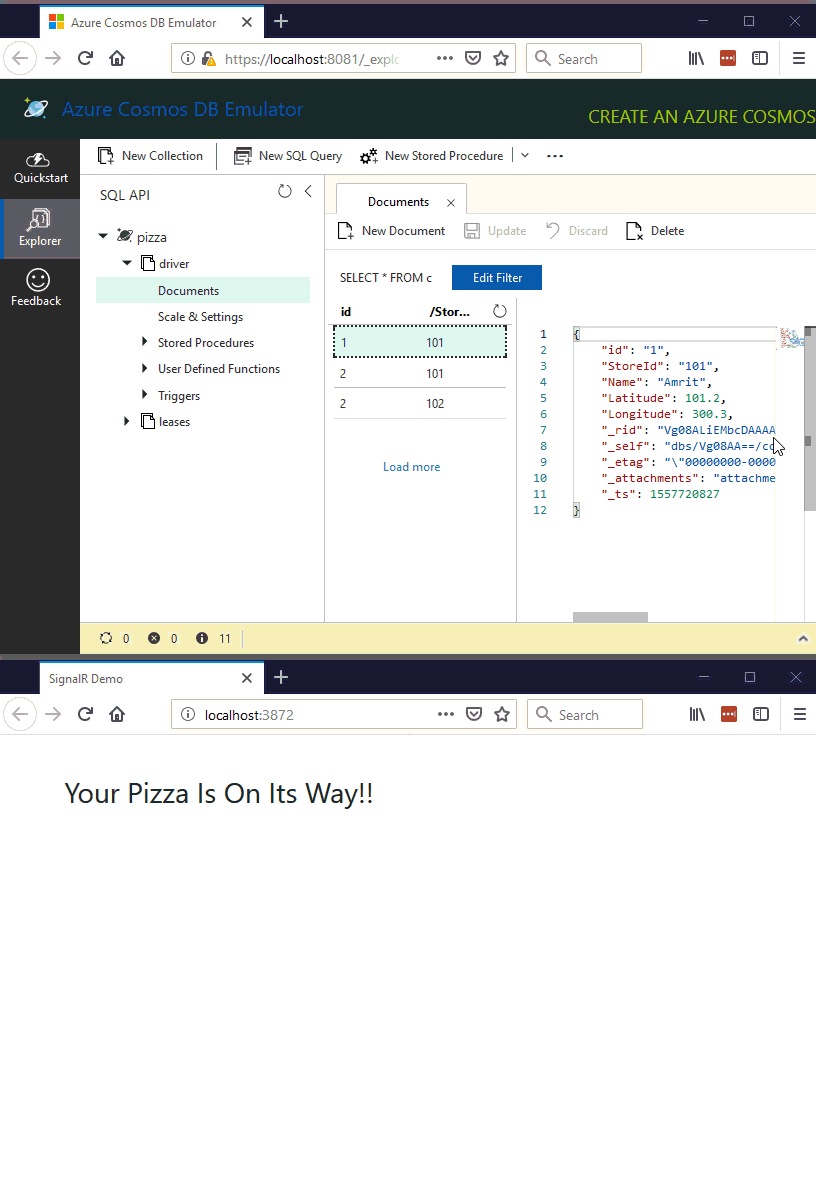
If you want to learn more about how to setup the SignalR Service, check out this previous article.
In this series we’ve been using the domain of pizza delivery. In this article we’ll see how updates in Cosmos DB can trigger a notification on an HTML client – you may have seen this if you’ve ordered pizza online where the delivery driver is tracked by GPS on a map.
The first thing to do is set up an Azure SignalR Service in Azure and add the SignalR Service connection string in the local.settings.json file which was covered in this article.
We can now add the negotiate function:
[FunctionName("negotiate")]
public static SignalRConnectionInfo GetDriverLocationUpdatesSignalRInfo(
[HttpTrigger(AuthorizationLevel.Anonymous, "post")] HttpRequest req,
[SignalRConnectionInfo(HubName = "driverlocationnotifications")] SignalRConnectionInfo connectionInfo)
{
return connectionInfo;
}
The preceding code sets the SignalR hub to be used in this example as “driverlocationnotifications”, we’ll use the same hub name in the function that actually sends updates to clients.
The next thing to do is add a function that is triggered when changes are made to the location of pizza delivery drivers (note the trigger will also fire for new documents that are added):
[FunctionName("PizzaDriverLocationUpdated")]
public static async Task Run([CosmosDBTrigger(
databaseName: "pizza",
collectionName: "driver",
ConnectionStringSetting = "pizzaConnection")] IReadOnlyList<Document> modifiedDrivers,
[SignalR(HubName = "driverlocationnotifications")] IAsyncCollector<SignalRMessage> signalRMessages,
ILogger log)
{
if (modifiedDrivers != null)
{
log.LogInformation($"Total modified drivers: {modifiedDrivers.Count}");
foreach (var modifiedDriver in modifiedDrivers)
{
var driverName = modifiedDriver.GetPropertyValue<string>("Name");
var driverLat = modifiedDriver.GetPropertyValue<double>("Latitude");
var driverLong = modifiedDriver.GetPropertyValue<double>("Longitude");
log.LogInformation($"Driver {modifiedDriver.Id} {driverName} was updated (lat,long) {driverLat}, {driverLong}");
var message = new DriverLocationUpdatedMessage
{
DriverId = modifiedDriver.Id,
DriverName = driverName,
Latitude = driverLat,
Longitude = driverLong
};
await signalRMessages.AddAsync(new SignalRMessage
{
Target = "driverLocationUpdated",
Arguments = new[] { message }
});
}
}
}
The preceding code uses the [CosmosDBTrigger] to fire the function when there are changes made to the “driver” collection in the “pizza” database.
The [SignalR] binding attribute allows outgoing SignalR messages to be sent to connected clients by adding messages to the IAsyncCollector<SignalRMessage> signalRMessages object. Notice that the type of the Cosmos DB trigger is IReadOnlyList<Document>, to get the actual data items of the document we use the GetPropertyValue method, for example to get the driver name: modifiedDriver.GetPropertyValue<string>("Name").
In the HTML, we can connect to the “driverlocationnotifications” SignalR hub, and then get notifications when any driver location changes, in reality we would probably only want to get messages for the driver that is delivering our pizza but for simplicity we won’t worry about it in this demo code.
The following is the HTML to make this work:
<html>
<head>
<!--Adapted from: https://azure-samples.github.io/signalr-service-quickstart-serverless-chat/demo/chat-v2/ -->
<title>SignalR Demo</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.1.3/dist/css/bootstrap.min.css">
<style>
.slide-fade-enter-active, .slide-fade-leave-active {
transition: all 1s ease;
}
.slide-fade-enter, .slide-fade-leave-to {
height: 0px;
overflow-y: hidden;
opacity: 0;
}
</style>
</head>
<body>
<p> </p>
<div id="app" class="container">
<h3>Your Pizza Is On Its Way!!</h3>
<div class="row" v-if="!ready">
<div class="col-sm">
<div>Loading...</div>
</div>
</div>
<div v-if="ready">
<transition-group name="slide-fade" tag="div">
<div class="row" v-for="driver in drivers" v-bind:key="driver.DriverId">
<div class="col-sm">
<hr />
<div>
<div style="display: inline-block; padding-left: 12px;">
<div>
<span class="text-info small"><strong>{{ driver.DriverName }}</strong> just changed location:</span>
</div>
<div>
{{driver.Latitude}},{{driver.Longitude}}
</div>
</div>
</div>
</div>
</div>
</transition-group>
</div>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue@2.5.17/dist/vue.js"></script>
<script src="https://cdn.jsdelivr.net/npm/@aspnet/signalr@1.1.2/dist/browser/signalr.js"></script>
<script src="https://cdn.jsdelivr.net/npm/axios@0.18.0/dist/axios.min.js"></script>
<script>
const data = {
drivers: [],
ready: false
};
const app = new Vue({
el: '#app',
data: data,
methods: {
}
});
const connection = new signalR.HubConnectionBuilder()
.withUrl('http://localhost:7071/api')
.configureLogging(signalR.LogLevel.Information)
.build();
connection.on('driverLocationUpdated', driverLocationUpdated);
connection.onclose(() => console.log('disconnected'));
console.log('connecting...');
connection.start()
.then(() => data.ready = true)
.catch(console.error);
let counter = 0;
function driverLocationUpdated(driverLocationUpdatedMessage) {
driverLocationUpdatedMessage.id = counter++; // vue transitions need an id
data.drivers.unshift(driverLocationUpdatedMessage);
}
</script>
</body>
</html>
Now when changes are made to the documents in the driver collection, the Azure Function will execute and send out Azure SignalR Service messages to clients. In this example we’re just writing out the messages in the page, but you can image the latitude and longitude being used to draw a little car image on top of a map, etc.
If you want to fill in the gaps in your C# knowledge be sure to check out my C# Tips and Traps training course from Pluralsight – get started with a free trial.


SHARE: