This is the first part in a series of articles.
Azure Cosmos DB is a “globally distributed, multi-model database service. With a click of a button, Cosmos DB enables you to elastically and independently scale throughput and storage across any number of Azure regions worldwide. You can elastically scale throughput and storage, and take advantage of fast, single-digit-millisecond data access using your favorite API including SQL, MongoDB, Cassandra, Tables, or Gremlin” [Microsoft]
One way to respond to changes in Cosmos is to use Azure Functions. When changes occur (currently limited to inserts and updates, e.g. not deletions ) the Function can be notified and executes.
Azure Function Cosmos DB triggers under the hood make use of the Azure Cosmos DB change feed to know when to execute functions.
Installing the Azure Cosmos Emulator
You can use the Azure Cosmos Emulator to get started locally without even needing an Azure subscription.
Once the emulator is downloaded and installed on your development PC (a Docker version is also available) you can start the emulator from the start menu.
When running you can see the icon in the Windows taskbar notification area and it will pop up a browser window pointing to https://localhost:8081/_explorer/index.html as the following screenshot shows:
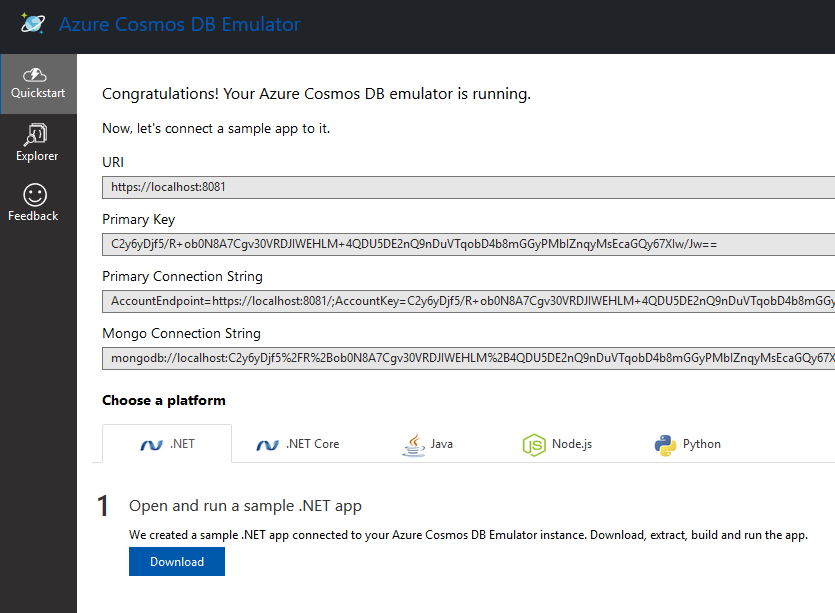
Notice in the preceding screenshot the Primary Connection String, you will need to copy this for use in the local app settings later.
Creating an Azure Function Triggered from Cosmos DB
Once you have created an Azure Function project in Visual Studio, you need to add the Microsoft.Azure.WebJobs.Extensions.CosmosDB NuGet package to get access to the .NET C# bindings.
Now the package is installed (and with an up to date installation of Visual Studio) you can right click the Azure Functions project and choose Add –> New Azure Function…
Give the function a name and for the trigger type choose Cosmos - this will create a boiler plate function like the following:
public static class PizzaDriverLocationUpdated
{
[FunctionName("PizzaDriverLocationUpdated")]
public static void Run([CosmosDBTrigger(
databaseName: "databaseName",
collectionName: "collectionName",
ConnectionStringSetting = "",
LeaseCollectionName = "leases")]IReadOnlyList<Document> input, ILogger log)
{
if (input != null && input.Count > 0)
{
log.LogInformation("Documents modified " + input.Count);
log.LogInformation("First document Id " + input[0].Id);
}
}
}
The preceding code uses the [CosmosDBTrigger] attribute to tell this function to execute when there are changes as specified by the following attribute parameters/properties:
- databaseName - Azure Cosmos DB database with the monitored collection
- collectionName – Collection being monitored for changes
- ConnectionStringSetting – App setting name containing the connection string to the Azure Cosmos DB being monitored
- LeaseCollectionName - name of the collection used to store leases, defaults to “leases” if not specified
The LeaseCollectionName is required by the trigger to store leases over Cosmos DB partitions, one thing to note: “If multiple functions are configured to use a Cosmos DB trigger for the same collection, each of the functions should use a dedicated lease collection or specify a different LeaseCollectionPrefix
for each function. Otherwise, only one of the functions will be triggered” [Microsoft]
How to Create a Database and Collection in the Azure Cosmos Emulator
In the emulator portal in the browser, click Explorer.This will allow you to create collections in the emulator.
Click the New Collection button and enter the following:
- Database id: pizza
- Collection Id: driverLocation
- Partition key: /storeId
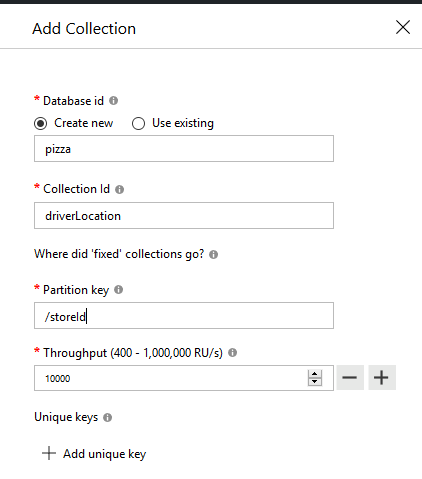
Save this new collection.
Configuring an Azure Function Cosmos DB Trigger
Modify the function that was created earlier to be as follows:
public static class PizzaDriverLocationUpdated
{
[FunctionName("PizzaDriverLocationUpdated")]
public static void Run([CosmosDBTrigger(
databaseName: "pizza",
collectionName: "driverLocation",
ConnectionStringSetting = "pizzaConnection")] IReadOnlyList<Document> input,
ILogger log)
{
if (input != null && input.Count > 0)
{
log.LogInformation("Documents modified " + input.Count);
log.LogInformation("First document Id " + input[0].Id);
}
}
}
Notice in the preceding code that the databaseName and collectionName settings match what we just created in the emulator.
The ConnectionStringSetting is set to “pizzaConnection” – this needs to be in the function settings, in the case of local development in the local.settings.json file:
{
"IsEncrypted": false,
"Values": {
"AzureWebJobsStorage": "UseDevelopmentStorage=true",
"FUNCTIONS_WORKER_RUNTIME": "dotnet",
"pizzaConnection": "AccountEndpoint=https://localhost:8081/;AccountKey=C2y6yDjf5/R+ob0N8A7Cgv30VRDJIWEHLM+4QDU5DE2nQ9nDuVTqobD4b8mGGyPMbIZnqyMsEcaGQy67XIw/Jw=="
},
"Host": {
"LocalHttpPort": 7071,
"CORS": "http://localhost:3872",
"CORSCredentials": true
}
}
Notice in the preceding settings, the value for the pizzaConnection item is the Primary Connection String copied from the Azure Cosmos Emulator.
Testing the Function Locally
Now that the function code is configured and the database and collection exist in the emulator, hit F5 in Visual Studio (or click Run) and the local functions runtime will start.
Once the runtime has started, head back to the Cosmos Emulator in the browser, click on Pizza –> driverLocation –> Documents and click the New Document button.
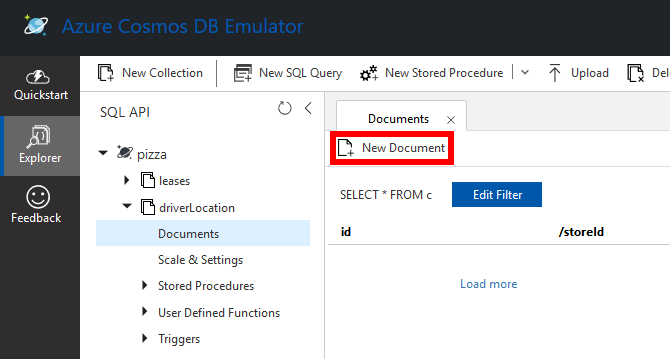
Add the following:
{
"id": "1",
"storeId": 42,
"name": "Sarah",
"lat" : 52,
"long": 2
}
And click Save.
Head back to the locally running functions runtime window and you will see the function has noticed this new document and executed:
Executing 'PizzaDriverLocationUpdated' (Reason='New changes on collection driverLocation at 2019-05-10T04:10:29.9913171Z', Id=203ae791-c7d4-4270-ac6b-501f313c3805)
Documents modified 1
First document Id 1
Executed 'PizzaDriverLocationUpdated' (Succeeded, Id=203ae791-c7d4-4270-ac6b-501f313c3805)
If you head back to the emulator, modify the document (e.g. change the name to “Amrit”) and click Update, the function will trigger a second time:
Executing 'PizzaDriverLocationUpdated' (Reason='New changes on collection driverLocation at 2019-05-10T04:13:00.8233214Z', Id=778caddd-9f19-42fa-9d9d-6c5dd5892a25)
Documents modified 1
First document Id 1
Executed 'PizzaDriverLocationUpdated' (Succeeded, Id=778caddd-9f19-42fa-9d9d-6c5dd5892a25)
If you want to fill in the gaps in your C# knowledge be sure to check out my C# Tips and Traps training course from Pluralsight – get started with a free trial.


SHARE: