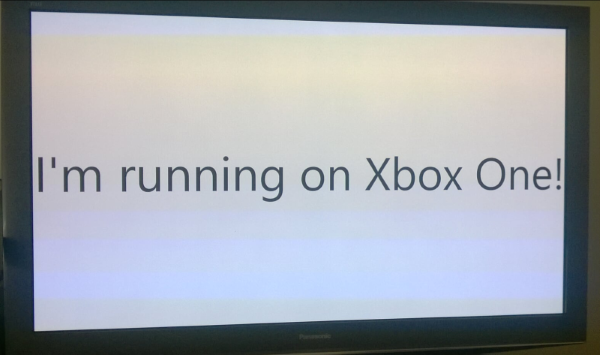
There’s been a number of almost-goosebump-inspiring moments during my .NET dev experience such as the first time I saw my code running on a Windows Phone 7. Another one of these moments was seeing my code running on my Xbox One for the first time.
(Note: this post describes pre-release technology)
It is now possible to take your regular Fallout 4 playing retail Xbox One and turn it into a development machine. This allows the running of Universal Windows Platform (UWP) apps. At the time of writing this is in preview/pre-release status with a final release expected later this year.
There’s a great set of documentation on MSDN that describes the following steps in detail. I’d recommend reading through them all before starting the process as there’s a number of warnings that should be observed before starting. For example “some popular games and apps will not work as expected, and you may experience occasional crashes and data loss. If you leave the developer preview, your console will factory reset and you will have to reinstall all of your games, apps, and content” [MSDN].
Also be aware that, to enable Xbox UWP app development in Visual Studio, the Windows 10 SDK preview build 14295 needs to be installed: “Installing this preview SDK on your PC will prevent you from submitting apps to the store built on this PC, so don’t do this on your production development PC” [MSDN]. I created a new Hyper-V virtual machine so as not to disturb my base machine.
The documentation recommends using a hardwired network connection rather than wireless for better dev performance, I used wireless and for this simple app and it was fine. Also note “…system performance in this preview does not reflect system performance of the final release” [MSDN].
Also note that you don’t need the latest Windows 10 preview build to install the tools, the virtual machine I created was just running standard Windows 10 Pro, though as the following screenshot shows this seems to mean that there is no XAML visual preview in Visual Studio.
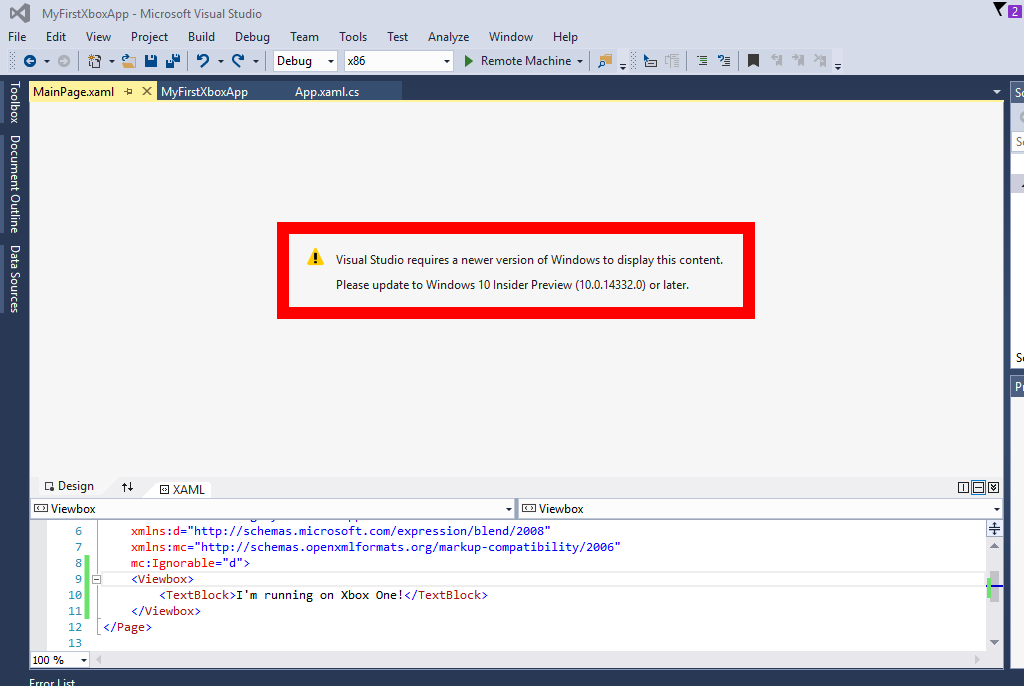
Overview of Steps
The following is an overview of the main steps required, once again you should consult MSDN for full details/steps required/warnings.
Step 1: Development Environment Setup
[MSDN]
You need:
- Visual Studio 2015 Update 2 or newer (be sure to install the Universal Windows App Development Tools component)
- Windows 10 SDK preview build 14295
- Sign up for Windows Insider program
- Create Windows Dev Center account
- Network connection to your Xbox One
Step 2: Xbox One Setup
Detailed steps from MSDN.
Sign in to Xbox One.
Install Dev Mode Activation app from Xbox One store
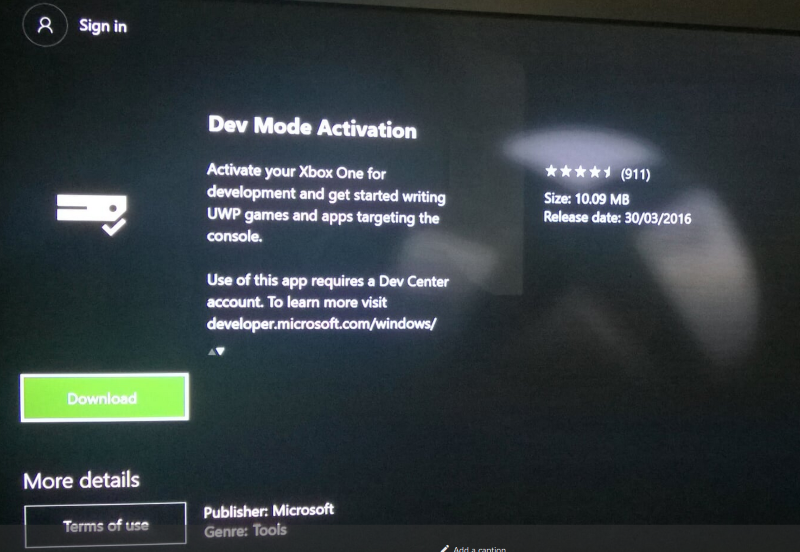
Once installed run the app:
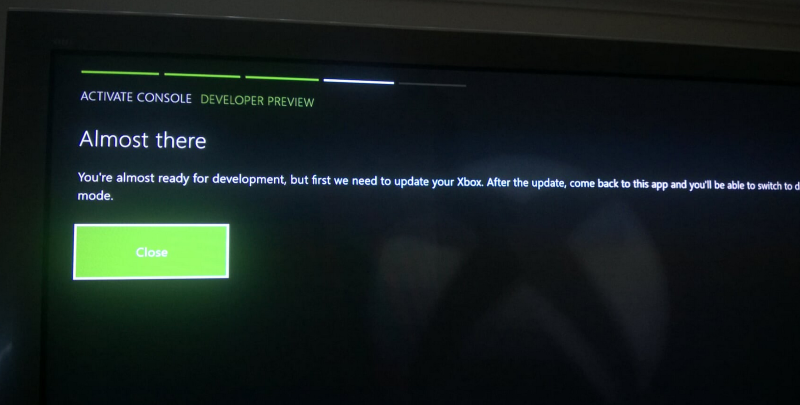
This can be a bit confusing as to what to do next, basically just leave it alone, at some point (perhaps hours) an “Update your Xbox” prompt will be displayed. Install the update and wait for it to complete and your Xbox restarted.
Open the Dev Mode Activation app again and following the instructions, to switch your console to into dev mode:
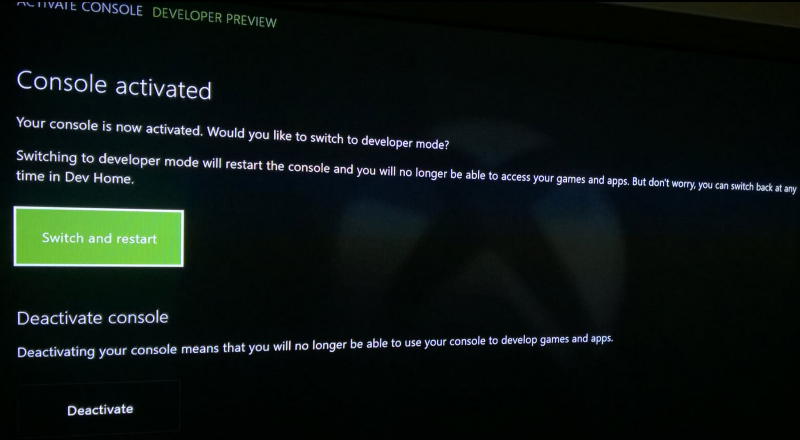
Tip: Make sure you’re connected to your wireless network before continuing…
Once restart is complete, open the Dev Home app:
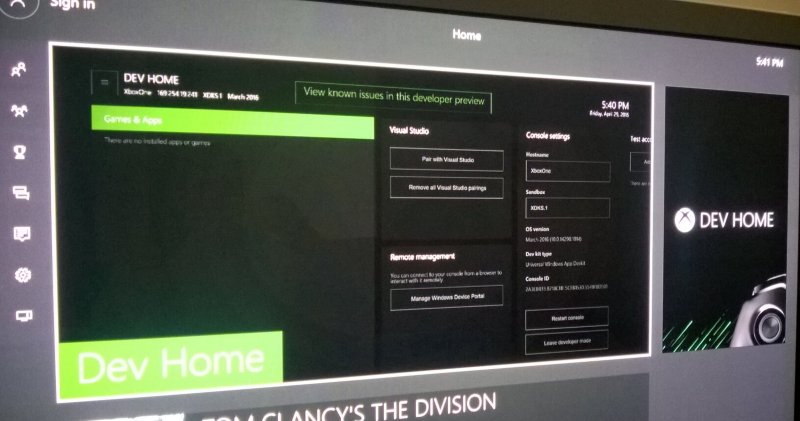
Take a note of the Xbox One’s IP address:
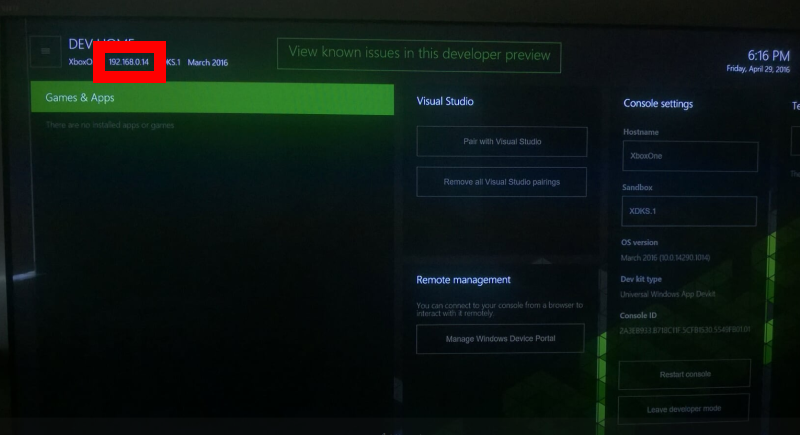
Step 3: Connection to Your Xbox One from Visual Studio
Create a new UWP project in Visual Studio.
Open the project properties and choose Remote Machine, enter the Xbox One’s IP address, and choose Universal (Unencrypted protocol).
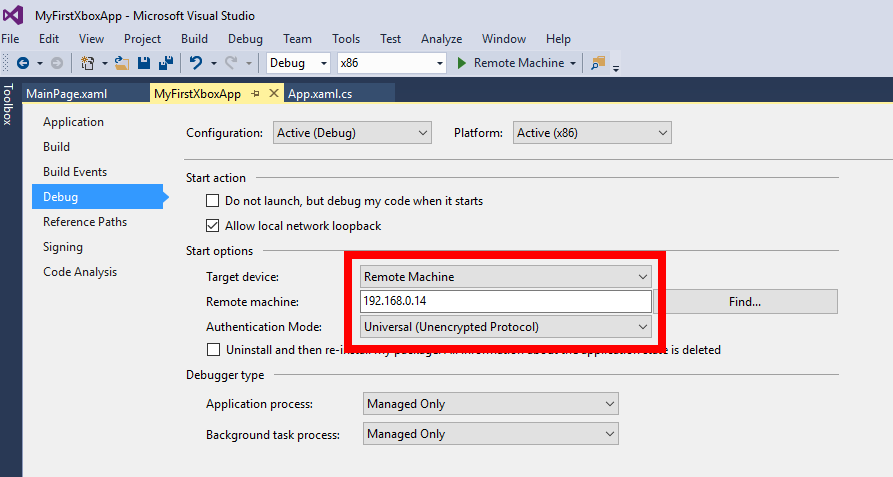
Next run the app, and Visual Studio will ask you for a PIN, head back to the Xbox One dev app, and choose “Pair with Visual Studio”, you’ll be given a PIN that you can type into Visual Studio.
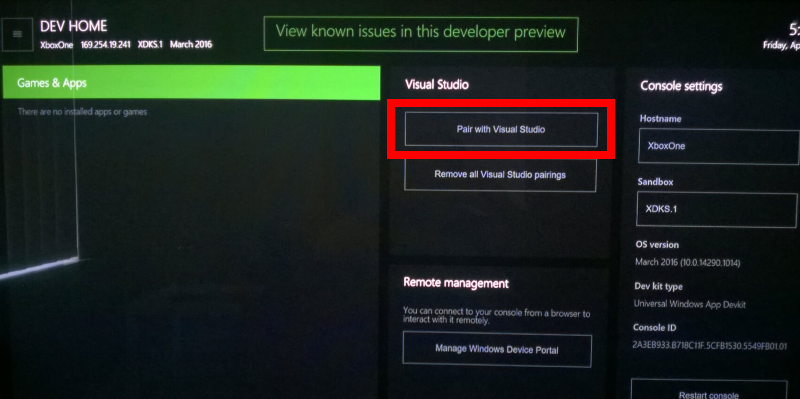
Your app should now be installed and run on your Xbox One!
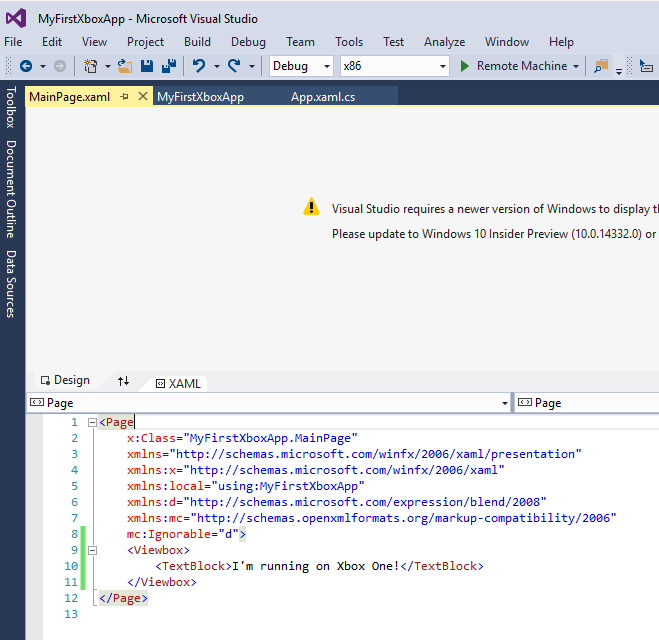
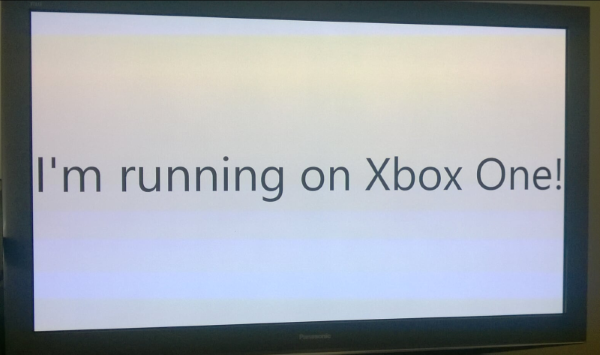
SHARE: