When using compiled data bindings using the x:Bind syntax in UWP apps, as an alternative to using an IValueConverter, a function can instead be defined. This means that whenever a value needs converting from the source object to the XAML property, the function will be called rather than an IValueConverter. This functionality is available from the Windows 10 Anniversary update so your UWP app in Visual Studio will need to be targeting version 14393 or later.
As an example, suppose there was already some existing code that maps whether a customer is considered high risk to a color, e.g. high risk customers should be shown in red. One way to accomplish this when databinding in the UI is to create an IValueConverter and then call into the existing code to do the conversion. Another option is to simply call the function from the XAML databinding – this reduces the need to write the additional IValueConverter implementation.
Take the following XAML:
<Page
x:Class="App1.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:App1"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<StackPanel>
<TextBlock Text="{x:Bind CustomerName}" FontSize="36"></TextBlock>
<TextBlock Text="{x:Bind IsHighRisk}" FontSize="36"></TextBlock>
</StackPanel>
</Grid>
</Page>
In the preceding XAML, we want the IsHighRisk TextBlock to appear in red if the customer is a high risk customer.
Imagine we already had some code to do this as follows:
using Windows.UI;
using Windows.UI.Xaml.Media;
namespace App1
{
static class RiskColorMapper
{
public static Brush ToColor(bool isHighRisk)
=> isHighRisk ? new SolidColorBrush(Colors.Red) : new SolidColorBrush(Colors.Olive);
}
}
We can now use the x:Bind syntax to call into this function and bind the foreground color to the boolean value of the IsHighRisk property as the following modified XAML demonstrates:
<Page
x:Class="App1.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:App1"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<StackPanel>
<TextBlock Text="{x:Bind CustomerName}" FontSize="36"></TextBlock>
<TextBlock Text="{x:Bind IsHighRisk}" FontSize="36" Foreground="{x:Bind local:RiskColorMapper.ToColor(IsHighRisk)}"></TextBlock>
</StackPanel>
</Grid>
</Page>
Now if we run the app with IsHighRisk set to false the text foreground is olive, if IsHighRisk is true the text will be red as the following screenshots show:
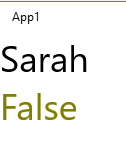
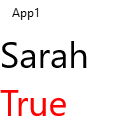
If the binding is TwoWay, the BindBack binding property can also be added that points to a function to do the reverse conversion.
For more info check out MSDN.
SHARE: