Windows 8.1 allows users to resize Windows more freely than Windows 8.0.
In Windows 8.0 there was the concept of snapped, filled and full screen viewstates. These concepts are no more in Windows 8.1 apps.
By default, Windows 8.1 apps have a minimum default width of 500px wide. This can be overridden and changed to 320px wide by setting it in the Package.appxmanifest file:
<ApplicationView MinWidth="width320" />
You can also use the designer:
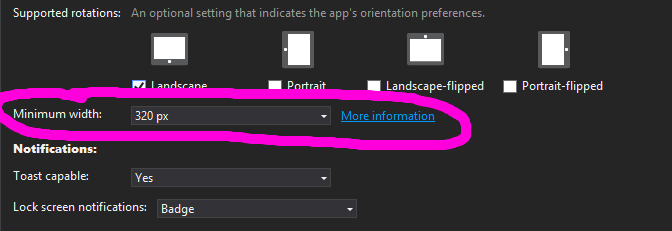
Depending on how you designed your app you may have more or less work to do; for example if you used a lot of fixed widths and positions you may have to adopt a more “responsive” layout strategy. If your existing app is more or less responsive already then you’ll probably have less work to do.
Initial Tests
Once you’ve decided if you’re going to stick with the default minimum 500px width or not, run your app and just see how it behaves at arbitrary widths in Windows 8.1. Pay attention to things that used to appear, disappear or change size with the old view state modes. Also test things such as settings flyouts still work correctly at the minimum window width.
For a basic checklist, test the following at different sizes, including the minimum width:
- App bar buttons (including context sensitive buttons, e.g. when item selected)
- Settings flyouts
- Lists, wrap panels, etc.
- Modal popup dialogs
- Page title
- Page back button
- Ad placement/size/visibility
Make a note of any problems that need fixing.
Debugging Widths
The next step is to decide what UI elements will change at different widths.
To help debugging widths, add the following XAML to your root layout Grid:
<TextBlock Name="DebugWidth"></TextBlock>
Next, add a SizeChanged event to your page, so it could look something like:
<LayoutAwarePage
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
SizeChanged="BasicPage1_OnSizeChanged">
And in the BasicPage1_OnSizeChanged event handler:
private void BasicPage1_OnSizeChanged(object sender, SizeChangedEventArgs e)
{
var w = Window.Current.Bounds.Width;
DebugWidth.Text = w.ToString();
}
Now whenever the window width changes, you’ll see the size updated in the in the TextBlock.
Once you’ve worked out what UI elements need to change at what sizes, you can use code in the OnSizeChanged event handler to change the UI state when certain widths are detected.
Changing Viewstates Based on Window Width
One possible way to deal with differing window widths is to define responsive breakpoints. These are those widths when the layout changes. Between each breakpoint size the app fluidly fills the available space.
One way to achieve this is to define some visual states / visual state groups in the page XAML, then switch to them in code when the window width crosses a breakpoint. For example, something like this:
private void SetViewStateFromWidth()
{
var w = Window.Current.Bounds.Width;
const int small = 410;
const int medium = 780;
const int large = 1180;
if (w < small)
{
VisualStateManager.GoToState(this, "S", false);
}
else if (w < medium)
{
VisualStateManager.GoToState(this, "M", false);
}
else if (w < large)
{
VisualStateManager.GoToState(this, "L", false);
}
else
{
VisualStateManager.GoToState(this, "Xl", false);
}
}
Here the view state switches between (S)mall, (M)edium, (L)arge, and extra large. The three consts are effectively the responsive breakpoints.
Final Tests
Once you’ve completed UI changes for different widths, run through the above checklist again and you can also run the app in the simulator at different screen sizes by clicking the screen icon in the simulator toolbar:
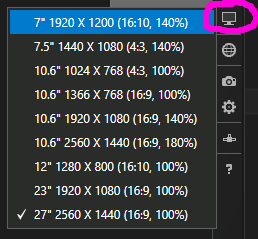
SHARE: