Microsoft Flow is a tool for creating workflows to automate tasks. It’s similar in concept to If This Then That but feels like it exists more towards the end of the spectrum of the business user rather than the end consumer – though both have a number of channels/services in common. Flow has a number of advanced features such as conditions, loops, timers, and delays.
Flow has a number of services including common ones such as Dropbox, OneDrive, Twitter, and Facebook. There are also generic services for calling HTTP services, including those created as Azure Functions. Essentially, services are the building blocks of a Flow.
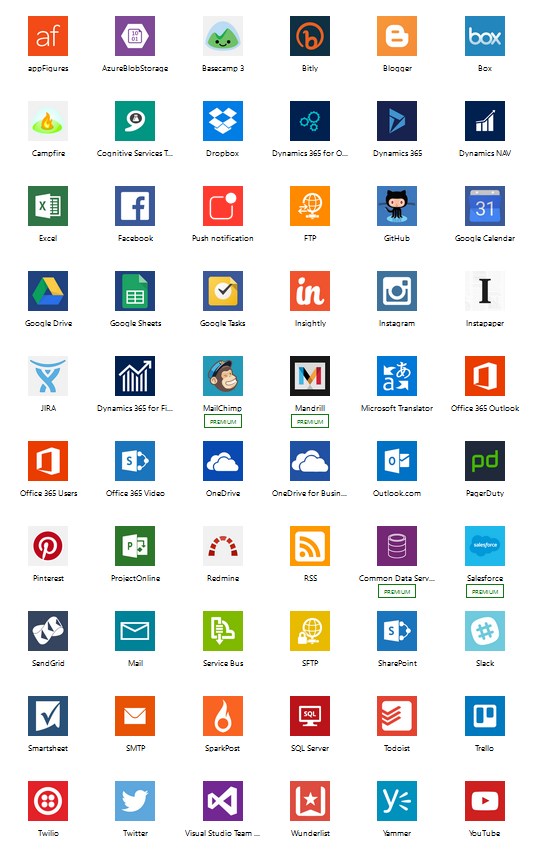
Once the free sign up is complete you can create Flows from existing templates or create your own from scratch.
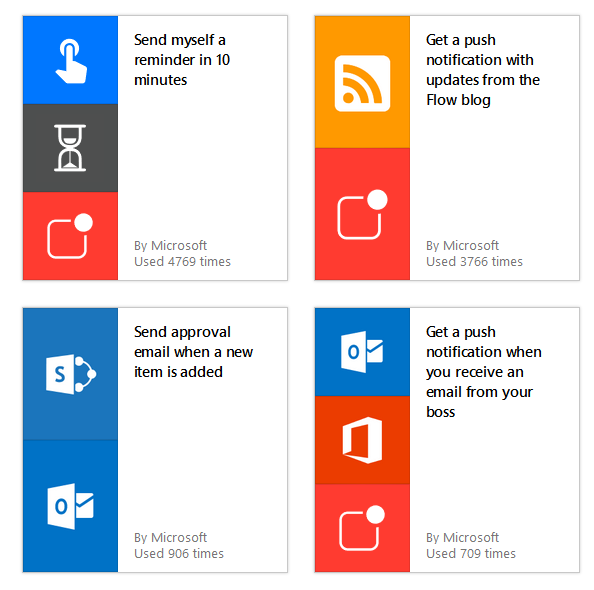
To create a new custom Flow, the web-based workflow designer can be used.
Integrating a Flow with Azure Functions
In the following example, a Flow will be created that picks up files with a specific naming convention from a OneDrive folder, sends the text content to an Azure Function that simply converts to uppercase and returns the result to the Flow. The Flow then writes out the uppercase version to another OneDrive folder.
Reading Files From OneDrive
The first step in the Flow is to monitor a specific OneDrive folder for new files.
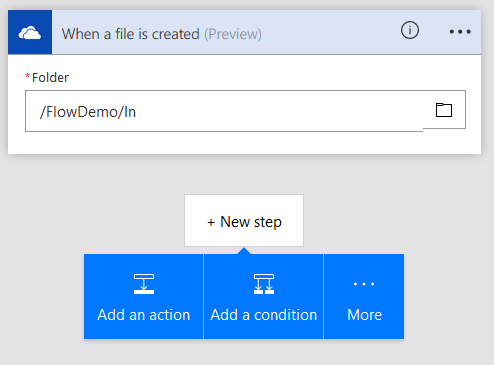
As an example of conditions, an “if statement” can be added to only process files that contain the word “data”:
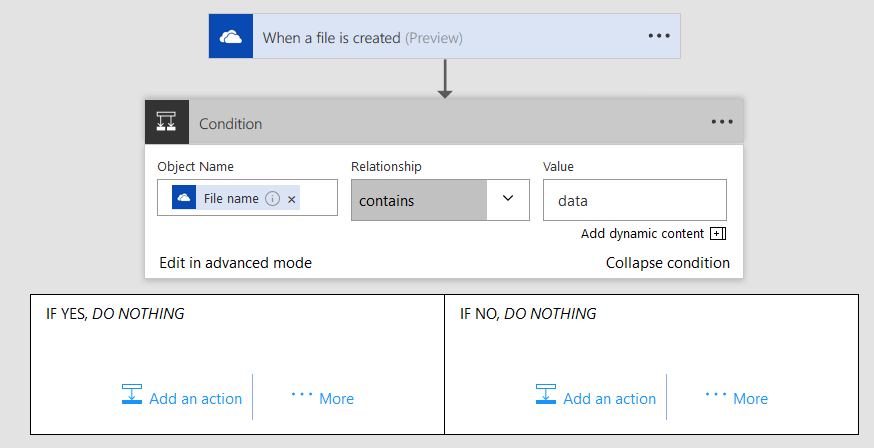
Now if the filename is correct we can go ahead and call an Azure Function (or other HTTP endpoint).
Calling an Azure Function from Microsoft Flow
Now that we are reading specific files, we want to call an Azure Function to convert the text content of the file to upper case.
The following code and screenshot shows the function that will be called – this code is stripped down and doesn’t contain any error checking/handling code for simplicity:
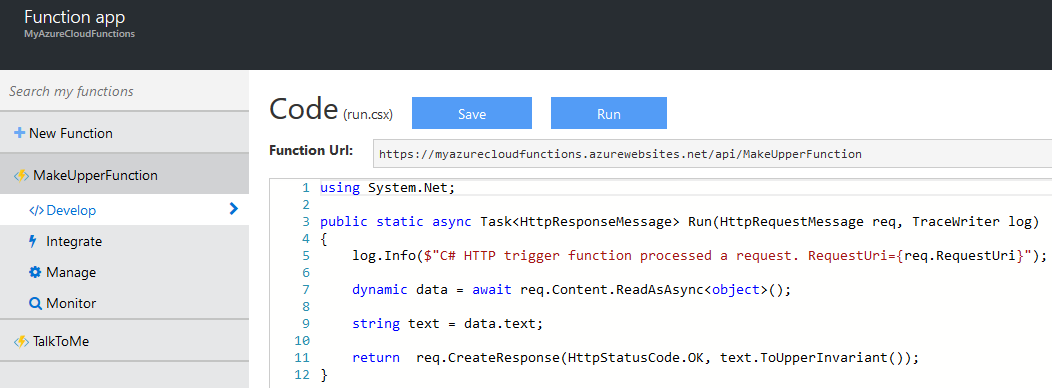
using System.Net;
public static async Task<HttpResponseMessage> Run(HttpRequestMessage req, TraceWriter log)
{
log.Info($"C# HTTP trigger function processed a request. RequestUri={req.RequestUri}");
dynamic data = await req.Content.ReadAsAsync<object>();
string text = data.text;
return req.CreateResponse(HttpStatusCode.OK, text.ToUpperInvariant());
}
We can test the API in Postman:
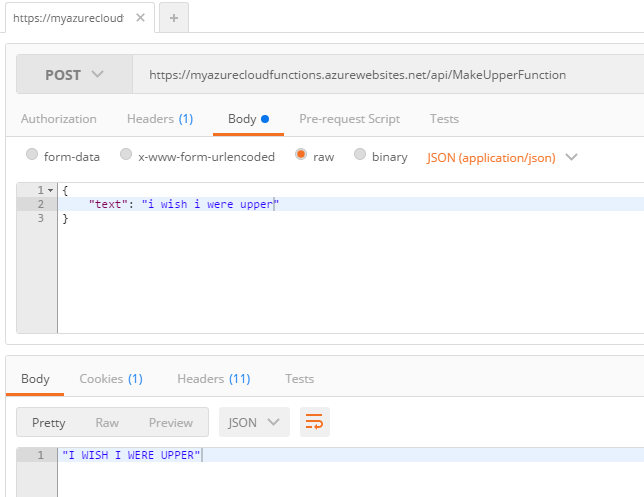
Now that we have a working function we can add a new action of type “HTTP” to the Flow and pass the contents of the OneDrive file as JSON data in the request. The final step is to take the response of calling the Azure Function and writing out to a new file in OneDrive as the following screenshot shows:
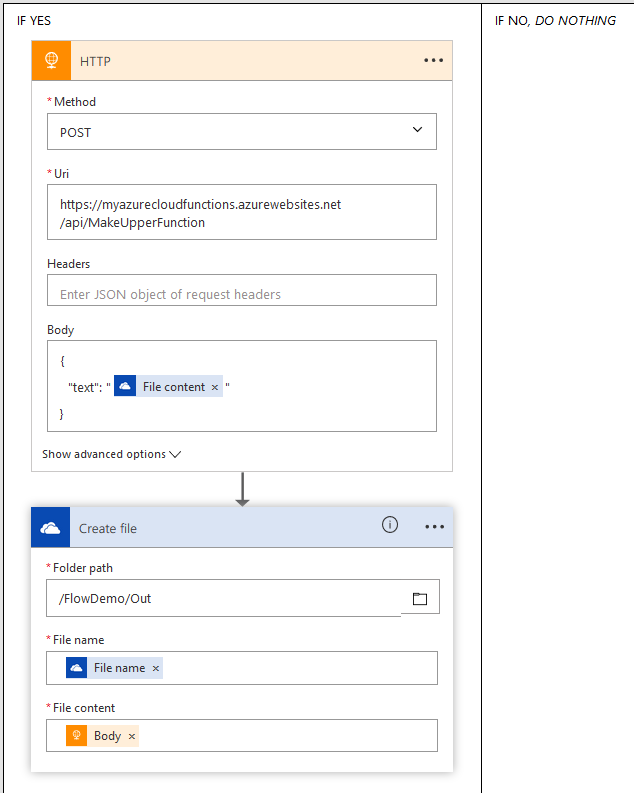
Now we can create a file “OneDrive\FlowDemo\In\test1data.txt”, the Flow will be trigged, and the output file “OneDrive\FlowDemo\Out\test1data.txt” created.
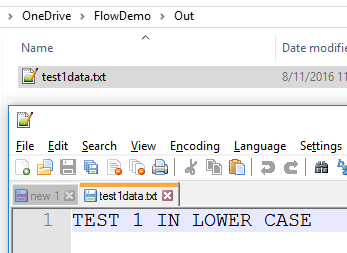
Microsoft Flow also has a really nice visual representation of runs (individual executions) of Flows:
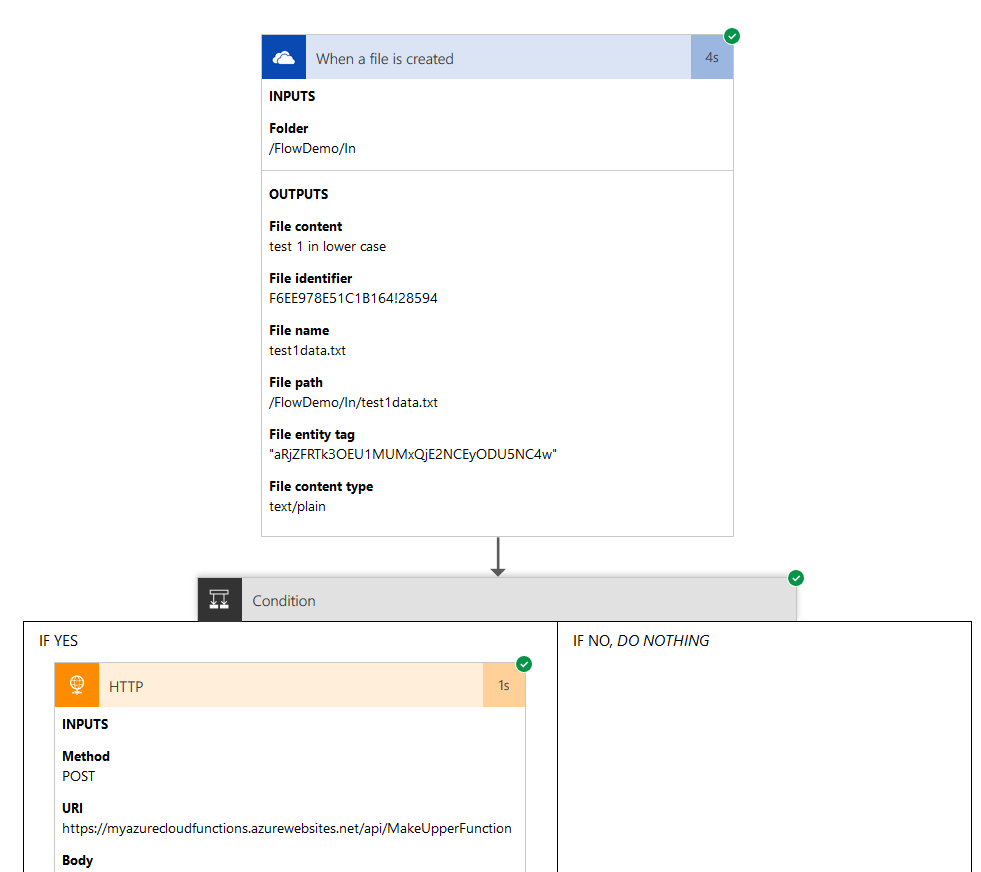
Microsoft Flow by itself enables a whole host of workflow scenarios, and combined with all the power of Azure Functions (and other Azure features) could enable some really interesting uses.
To jump-start your Azure Functions knowledge check out my Azure Function Triggers Quick Start Pluralsight course.
You can start watching with a Pluralsight free trial.


SHARE: