ASP.Net 4 allows us to use an opt-in viewstate approach rather than an opt-out approach in previous ASP.Net versions (EnableViewState was available in prior versions, but if you disable viewstate, all children of the page/container also inherited this with no one to override and opt-in).
To use the opt-in approach in ASP.Net 4, the new ViewStateMode property can be used. The following example disables viewstate for the whole page (all children will inherit this disabled value) but Label2 opts in to viewstate. The codebehind sets the values of the labels only on the first page load - not any subsequent postbacks.
<%@ Page Language="C#" AutoEventWireup="true"
CodeFile="Default.aspx.cs" Inherits="_Default"
ViewStateMode="Disabled"
%>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<p><asp:Label ID="Label1" Text="not set" runat="server" /></p>
<p><asp:Label ID="Label2" Text="not set" ViewStateMode="Enabled" runat="server" /></p>
<p><asp:Button Text="do a postback" runat="server" /></p>
</div>
</form>
</body>
</html>
Code behind:
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
Label1.Text = "this text has been set from code behind only on the first load i.e. not set in any following postbacks.";
Label2.Text = Label1.Text;
}
}
The first time the page loads, the 2 text boxes show the text as set in the code behind:
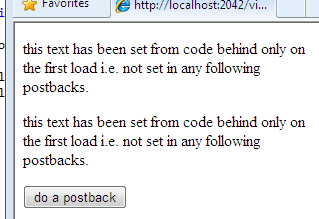
Once a postback happens (in this case by clicking the button), no code is executed in the Page_Load event due to the if (!IsPostBack) check. This means that the Text property of the labels will be set to what's initially declared in the aspx mark-up (i.e. "not set"); however because we have set ViewStateMode="Enabled" on Label2, it's Text will be retained in viewstate between callbacks:
SHARE: