If we have a longer running process taking place in a console application, it’s useful to be able to provide some feedback to the user so they know that the application hasn’t crashed. In a GUI application we’d use something like an animated progress bar or spinner. In a console application we can make use of the SetCursorPosition() method to keep the cursor in the same place while we output characters, to create a spinning animation.
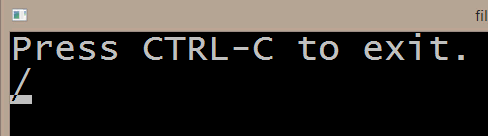
While the code below could certainly be improved, it illustrates the point:
using System;
namespace ConsoleDemo
{
internal class ConsoleSpinner
{
private int _currentAnimationFrame;
public ConsoleSpinner()
{
SpinnerAnimationFrames = new[]
{
'|',
'/',
'-',
'\\'
};
}
public char[] SpinnerAnimationFrames { get; set; }
public void UpdateProgress()
{
// Store the current position of the cursor
var originalX = Console.CursorLeft;
var originalY = Console.CursorTop;
// Write the next frame (character) in the spinner animation
Console.Write(SpinnerAnimationFrames[_currentAnimationFrame]);
// Keep looping around all the animation frames
_currentAnimationFrame++;
if (_currentAnimationFrame == SpinnerAnimationFrames.Length)
{
_currentAnimationFrame = 0;
}
// Restore cursor to original position
Console.SetCursorPosition(originalX, originalY);
}
}
}
using System;
using System.Threading;
namespace ConsoleDemo
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Press CTRL-C to exit.");
var s = new ConsoleSpinner();
while (true)
{
Thread.Sleep(100); // simulate some work being done
s.UpdateProgress();
}
}
}
}
Notice that in the animation, the cursor is still visible which looks a bit ugly. We can fix that with the CursorVisible property:
public void UpdateProgress()
{
// Hide cursor to improve the visuals (note: we should re-enable at some point)
Console.CursorVisible = false;
// Store the current position of the cursor
var originalX = Console.CursorLeft;
var originalY = Console.CursorTop;
// etc.
}
Now we get something nicer looking:
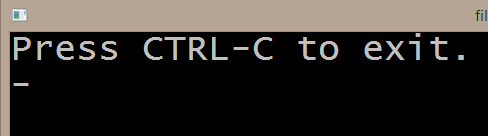
If we want different animation frames we can choose our own, the example below uses some Unicode characters:
var s = new ConsoleSpinner();
s.SpinnerAnimationFrames = new[]
{
'░',
'▒',
'▓'
};
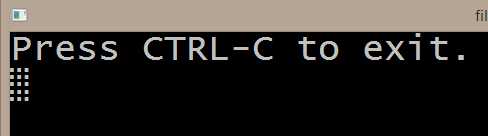
For more console related tips, check out my Building .NET Console Applications in C# Pluralsight course.
You can start watching with a Pluralsight free trial.


SHARE: