The Console class can do more than just WriteLine().
Here’s 3 fun/weird/useful/annoying things.
1. Setting The Console Window Size
The Console.SetWindowSize(numberColumns, numberRows) method sets the console window size.
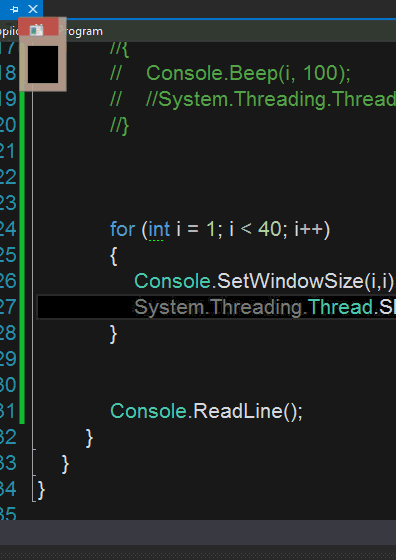
To annoy your users (or create a “nice” console opening animation) as this animated GIF shows you could write something like:
for (int i = 1; i < 40; i++)
{
Console.SetWindowSize(i,i);
System.Threading.Thread.Sleep(50);
}
2. Beeping Consoles
The Console.Beep() method emits a beep from the speaker(s).
We can also specify a frequency and duration.
The following console application allows the performer to adjust frequency and duration by using the keyboard arrows:
internal class Program
{
private static int _frequency = 10000;
private static int _duration = 100;
private static void Main(string[] args)
{
Console.WriteLine("Use keyboard arrows to adjust frequency and duration");
Console.WriteLine("Press CTRL-C to quit");
do
{
// While no one is pressing any keys just keep on beeping
while (!Console.KeyAvailable)
{
Console.Beep(_frequency, _duration);
}
// A key has been pressed, read what key (and prevent echoing to console output)
var k = Console.ReadKey(true);
switch (k.Key)
{
case ConsoleKey.UpArrow:
IncreaseFrequency();
break;
case ConsoleKey.DownArrow:
DecreaseFrequency();
break;
case ConsoleKey.RightArrow:
IncreaseDuration();
break;
case ConsoleKey.LeftArrow:
DecreaseDuration();
break;
}
} while (true);
}
private static void IncreaseDuration()
{
_duration += 100;
_duration = Math.Min(_duration, 1000);
}
private static void DecreaseDuration()
{
_duration -= 100;
_duration = Math.Max(_duration, 100);
}
private static void IncreaseFrequency()
{
_frequency += 100;
_frequency = Math.Min(_frequency, 15000);
}
private static void DecreaseFrequency()
{
_frequency -= 100;
_frequency = Math.Max(_frequency, 1000);
}
}
3. Fun With Titles
The Title property sets what appears in the console window title.
We can create an indeterminate style progress bar in the title by using a Unicode block character.
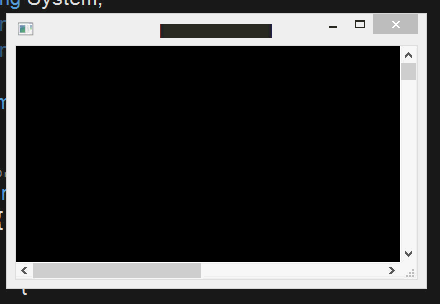
internal class Program
{
private static void Main(string[] args)
{
const int maxProgressBarLength = 10;
const string progressBarElement = "█";
var title = "";
do
{
title += progressBarElement;
if (title.Length > maxProgressBarLength)
{
title = progressBarElement;
}
Console.Title = title;
Thread.Sleep(100);
} while (true);
}
}
For more console related tips, check out my Building .NET Console Applications in C# Pluralsight course.
You can start watching with a Pluralsight free trial.


SHARE: