Azure functions allow the creation of HTTP-triggered code. A new feature to Functions is the ability to define proxies. (Note: at the time of writing this feature is in preview)
For example a function can be created that responds to HTTP GETs to retrieve a customer as the following code demonstrates:
using System.Net;
public class Customer
{
public int Id {get; set;}
public string Name {get; set;}
}
public static async Task<HttpResponseMessage> Run(HttpRequestMessage req, TraceWriter log)
{
// error checking omitted
string id = req.GetQueryNameValuePairs()
.FirstOrDefault(q => string.Compare(q.Key, "id", true) == 0)
.Value;
return req.CreateResponse(HttpStatusCode.OK, GetById(int.Parse(id)));
}
public static Customer GetById(int id)
{
// simulate fetching a customer, e.g. from Table storage, db, etc.
return new Customer
{
Id = id,
Name = "Amrit"
};
}
This function (called “GetCustomer”) could be available at the following URL (with function authorization enabled): https://dontcodetireddemos.azurewebsites.net/api/GetCustomer?id=42&code=rEQKsObGFDRCCiVuLhOnZ1Rdfn/XGgp5tfC1xHrhAqxvWqzHSQszCg==
Notice in the URL the name of the function is prefixed with “api” i.e. “api/GetCustomer”
Calling this URL would result in the following JSON being returned:
{
"Id": 42,
"Name": "Amrit"
}
Function proxies allow the “api” part to be replaced, for example to create a URL: “https://dontcodetireddemos.azurewebsites.net/customer?id=42&code=rEQKsObGFDRCCiVuLhOnZ1Rdfn/XGgp5tfC1xHrhAqxvWqzHSQszCg==”
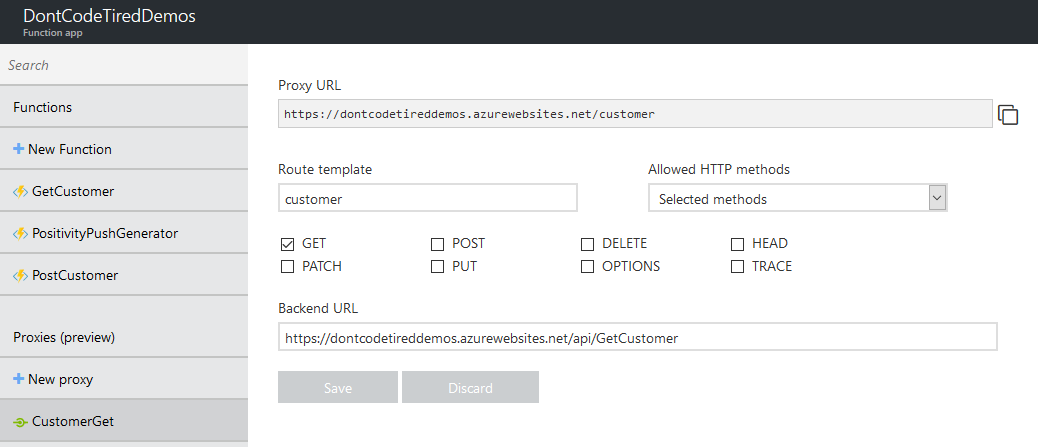
This proxy defines a name “CustomerGet”, a route template of “customer”, the actual URL to maps to “https://dontcodetireddemos.azurewebsites.net/api/GetCustomer”, and limited to only GET HTTP verbs.
If we had another function e.g. called PostCustomer we could also setup a POST proxy for that function:
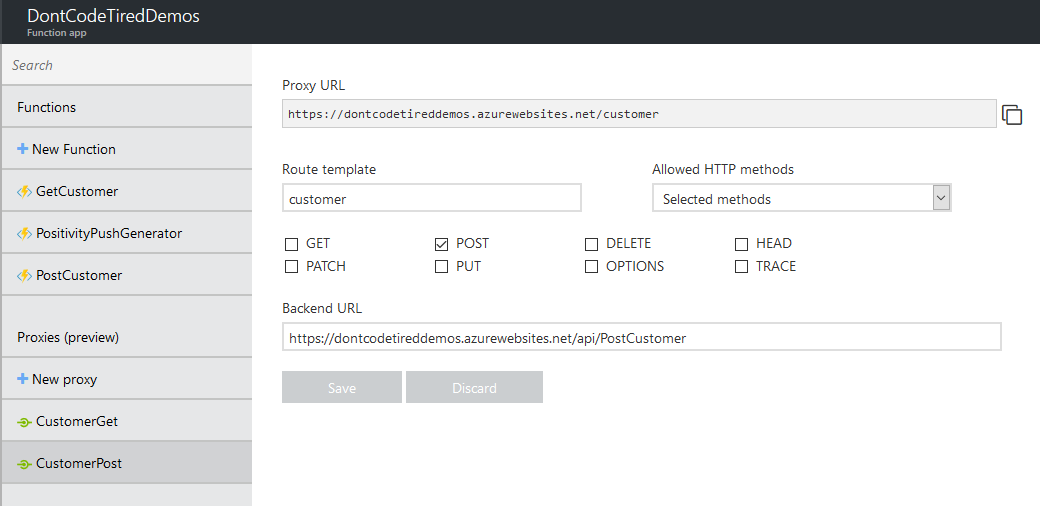
Now we can issue both POSTs and GETs to the customer “resource” at: https://dontcodetireddemos.azurewebsites.net/customer (code key omitted).
Using Kudu for example, we can see a proxies.js file created in the wwwroot folder with the following contents:
{
"proxies": {
"CustomerGet": {
"matchCondition": {
"route": "customer",
"methods": [
"GET"
]
},
"backendUri": "https://dontcodetireddemos.azurewebsites.net/api/GetCustomer"
},
"CustomerPost": {
"matchCondition": {
"route": "customer",
"methods": [
"POST"
]
},
"backendUri": "https://dontcodetireddemos.azurewebsites.net/api/PostCustomer"
}
}
}
To jump-start your Azure Functions knowledge check out my Azure Function Triggers Quick Start Pluralsight course.
SHARE: