In part 1 we created a Flow to toggle the sending of push notifications on and off by storing the configuration in Azure blob storage.
Now that we have a way of enabling/disabling notifications we can start to build the second Flow.
Before jumping into the Flow designer, we need to consider how to generate random positivity phrases and how to integrate this into the second Flow. One option to do this is to create a simple Azure Function with an HTTP trigger. The Flow can then use an HTTP action to issue a GET to the server that will return the string content to be sent via push notifications.
In the Azure Portal function editor a new function can be created with a HTTP trigger configured to GET only as the following screenshot shows:
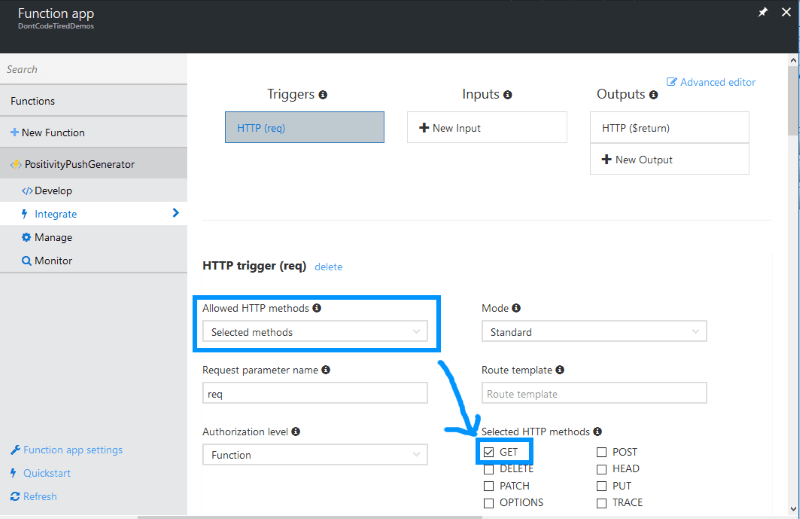
Notice in the preceding screenshot the authorization level has bee set to “function”. This means the key needs to be provided when the function is called.
We can now write some code in the function code editor window as follows:
using System.Net;
public static HttpResponseMessage Run(HttpRequestMessage req, TraceWriter log)
{
log.Info("C# HTTP trigger function processed a request.");
string phrase = GeneratePhrase();
return req.CreateResponse(HttpStatusCode.OK, phrase);
}
public static string GeneratePhrase()
{
var phrases = new string[]
{
"Don't worry, be happy :)",
"All is well",
"Will it matter in 100 years?",
"Change what you can, don't worry about what you can't"
};
var rnd = new Random();
return phrases[rnd.Next(phrases.Length)];
}
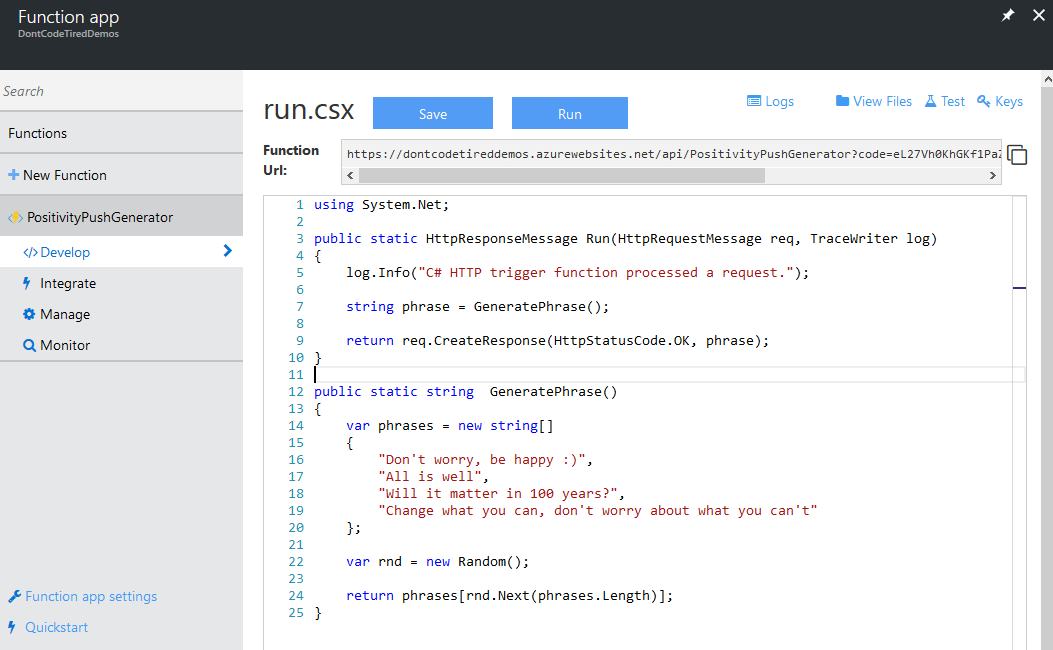
Clicking the Run button will test the function and we can see the random phrases being returned with a 200 status as shown in the following screenshot:
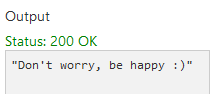
In the final part of this series we’ll go and create the second Flow that uses this function and the configuration value created in the previous article to actually send random positivity push notifications on a 15 minute schedule.
To jump-start your Azure Functions knowledge check out my Azure Function Triggers Quick Start Pluralsight course.
SHARE: