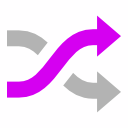
Version 3 of my open source feature toggle library is now released to NuGet.
Version 3 introduces some breaking changes and new features.
Breaking Changes
- The WPF value convertor package has been removed for version 3 as it’s trivial to implement a version in individual applications and reduce the maintenance overhead for the porject
- Version 3 removes support for Windows Phone 8.0 (you can still use version 2.x if you need this support)
New Features
Platforms Support
In addition to .NET framework 4 full, version 3 also supports:
- Windows Phone 8.1 Silverlight apps
- Windows Universal Apps 8.1 (Store and Windows Phone 8.1 WinRT)
- Windows Store 8.1 apps
Future versions will add support for Windows 10 apps.
New Toggle Types
The EnabledOnOrAfterAssemblyVersionWhereToggleIsDefinedToggle (a little verbose I know) allows a feature to become enabled once a specified assembly version number is reached. The assembly version used is that of the assembly inside which the toggle is defined, rather that the executing assembly. The configuration value for this toggle would be (for example) 0.0.2.1.
Future version will add support for comparing the executing assembly version.
New Decorators
A new type of feature that version 3 introduces is the idea of toggle decorators. These are classes that “wrap” one or more toggles and provide additional features.
The DefaultToEnabledOnErrorDecorator and the DefaultToDisabledOnErrorDecorator allows a default value to provided if there is an error evaluating the wrapped toggle value, for example with a configuration error. These decorators should be used with caution as your application may end up in an unknown state if you forget to configure something properly. In some cases you may want this behaviour rather than the application failing.
Assuming that a feature toggle called MyFeatureToggle has been defined, to use the decorator the following code would be used.
IFeatureToggle printFeatureThatDefaultsToDisabled = new DefaultToDisabledOnErrorDecorator( new MyFeatureToggle() );
bool isPrintEnabled = printFeatureThatDefaultsToDisabled.FeatureEnabled;
These decorators also allow you to specify an optional logging action that gets called if the wrapped toggle throws an exception:
new DefaultToDisabledOnErrorDecorator(wrappedToggle, ex => Console.WriteLine(ex.Message));
The CompositeAndDecorator allows multiple toggles to be wrapped, only if all these wrapped toggles are enabled will the decorator return true.
IFeatureToggle andToggle = new CompositeAndDecorator(new AnEnabledFeature(), new ADisabledFeature());
var isEnabled = andToggle.FeatureEnabled; // returns false
The final new decorator is the FixedTimeCacheDecorator. This allows a toggle that gets its value from a slow source (such as remote database, http, etc.) to have its value cached for a fixed period of time. To use this you specify the wrapped toggle and a TimeSpan.
IFeatureToggle cachedToggle = new FixedTimeCacheDecorator(cachedToggle, TimeSpan.FromSeconds(10));
Check out the release notes on GitHub. If you find any problems or bugs or want to suggest other features, please create an issue on GitHub.
SHARE: