To compliment the release of my Testing .NET Code with xUnit.net 2 Pluralsight course, I’ve updated my original xUnit.net cheat sheet.
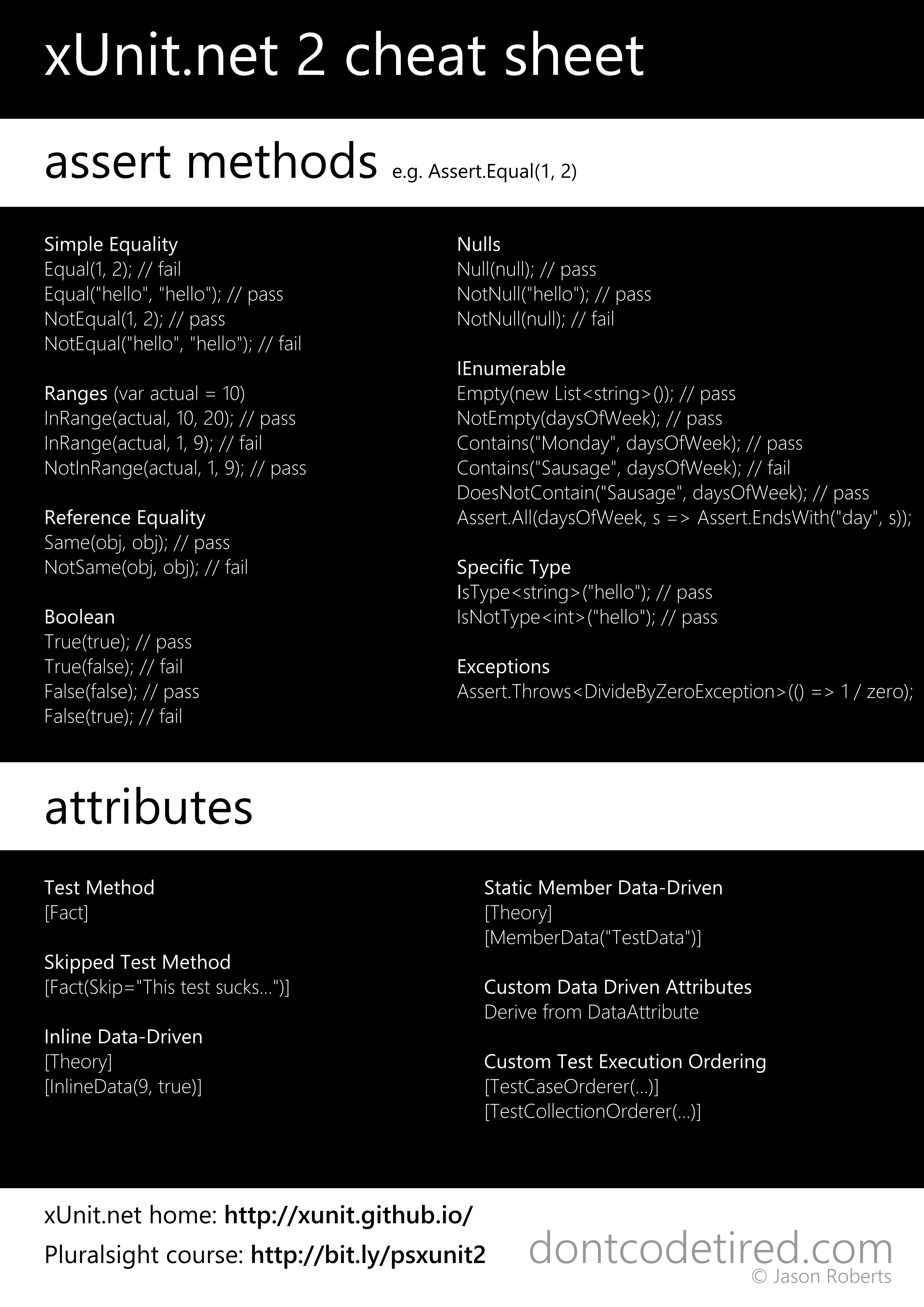
(To download just right-click, save-as)
To learn xUnit.net check out my xUnit.net Pluralsight training course.
You can start watching with a Pluralsight free trial.


SHARE:
xUnit.net allows the creation of data-driven tests. These kind of tests get their test data from outside the test method code via parameters added to the test method signature.
Say we had to test a Calculator class and check it’s add method returned the correct results for six different test cases. Without data-driven tests we’d either have to write six separates tests (with almost identical code) or some loop inside our test method containing an assert.
Regular xUnit.net test methods are identified by applying the [Fact] attribute. Data-driven tests instead use the [Theory] attribute.
To get data-driven features and the [Theory] attribute, install the xUnit.net Extensions NuGet package in addition to the standard xUnit.net package.
Creating Inline Data-Driven Tests
The [InlineData] attribute allows us to specify test data that gets passed to the parameters of test method.
So for our Calculator add test we’d start by defining the test method:
More...
SHARE:
To help people get started with xUnit.net and as an accompaniment to my Pluralsight xUnit.net training course I thought I’d create a cheat sheet showing common assert methods and attributes. Hopefully will be of use :)
More...
SHARE:
My latest Pluralsight course on the xUnit.net testing framework has just been released.
Course Description
Learn the latest in unit testing technology for C#, VB.NET (and other .NET languages) created by the original inventor of NUnit.
xUnit.net is a free, extensible, open source framework designed for programmers that aligns more closely with the .NET platform.
You can check it out now on Pluralsight.com.
You can start watching with a Pluralsight free trial.


SHARE:
The below is an excerpt from the latest chapter “An Introduction to Unit Testing With xUnit.net” from my book Keeping Software Soft.
xUnit.net provides a number of ways for checking (asserting) results are as expected.
The following explanatory tests shown the different types of assertions that xUnit.net supports:
using System;
using System.Collections.Generic;
using Xunit;
namespace KeepingSoftwareSoftSamples.XUnitTestingDemo
{
public class XUnitAssertExamples
{
[Fact]
public void SimpleAssertsThatOneValueEqualsAnother()
{
Assert.Equal(1, 2); // fail
Assert.Equal("hello", "hello"); // pass
Assert.NotEqual(1, 2); // pass
Assert.NotEqual("hello", "hello"); // fail
}
[Fact]
public void BooleanAsserts()
{
Assert.True(true); // pass
Assert.True(false); // fail
Assert.False(false); // pass
Assert.False(true); // fail
// Don't do this
Assert.True(1 == 1); // pass
}
[Fact]
public void Ranges()
{
const int value = 22;
Assert.InRange(value, 21, 100); // pass
Assert.InRange(value, 22, 100); // pass
Assert.NotInRange(value, 999, 99999); // pass
Assert.InRange(value, 23, 100); // fail
}
[Fact]
public void Nulls()
{
Assert.Null(null); // pass
Assert.NotNull("hello"); // pass
Assert.NotNull(null); // fail
}
[Fact]
public void ReferenceEquality()
{
var objectA = new Object();
var objectB = new Object();
Assert.Same(objectA, objectB); // fail
Assert.NotSame(objectA, objectB); // pass
}
[Fact]
public void AnIEnumberableContainsASpecificItem()
{
var days = new List<string>
{
"Monday",
"Tuesday"
};
Assert.Contains("Monday", days); // pass
Assert.Contains("Friday", days); // fail
Assert.DoesNotContain("Friday", days); // pass
}
[Fact]
public void IEnumerableEmptiness()
{
var aCollection = new List<string>();
Assert.Empty(aCollection); // pass
aCollection.Add("now no longer empty");
Assert.NotEmpty(aCollection); // pass
Assert.Empty(aCollection); // fail
}
[Fact]
public void IsASpecificType()
{
Assert.IsType<string>("hello"); // pass
Assert.IsNotType<int>("hello"); // pass
Assert.IsType<int>("hello"); // fail
}
[Fact]
public void IsAssignableFrom()
{
const string stringVariable = "42";
Assert.IsAssignableFrom<string>(stringVariable); // pass
Assert.IsAssignableFrom<int>(stringVariable); // fail
}
}
}
To learn more about xUnit.net check out my xUnit.net Pluralsight course.
You can start watching with a Pluralsight free trial.


SHARE:
Until xUnit officially supports* Windows store apps you can get xUnit working with your WinRT app by doing the following:
In Visual Studio 2012, go to Tools menu, Extensions and Updates; search for and install “xUnit.net runner for Visual Studio 2012”.

In Visual Studio 2012, go to Tools menu, Extensions and Updates; search for and install “xUnit Test Library Template”.

You may have to restart Visual Studio…
More...
SHARE: