With the release of Visual Studio 2017 update 3, the new C# 7.1 features became available.
To use the new features, the .csproj file can be modified and the <LangVersion> element set to either “latest” (the newest release including minor releases) or explicitly to “7.1” , for example:
<LangVersion>latest</LangVersion>
or
<LangVersion>7.1</LangVersion>
To select one of these in Visual Studio, go to the project properties and the build tab and choose the “Advanced…” button as the following screenshot shows:
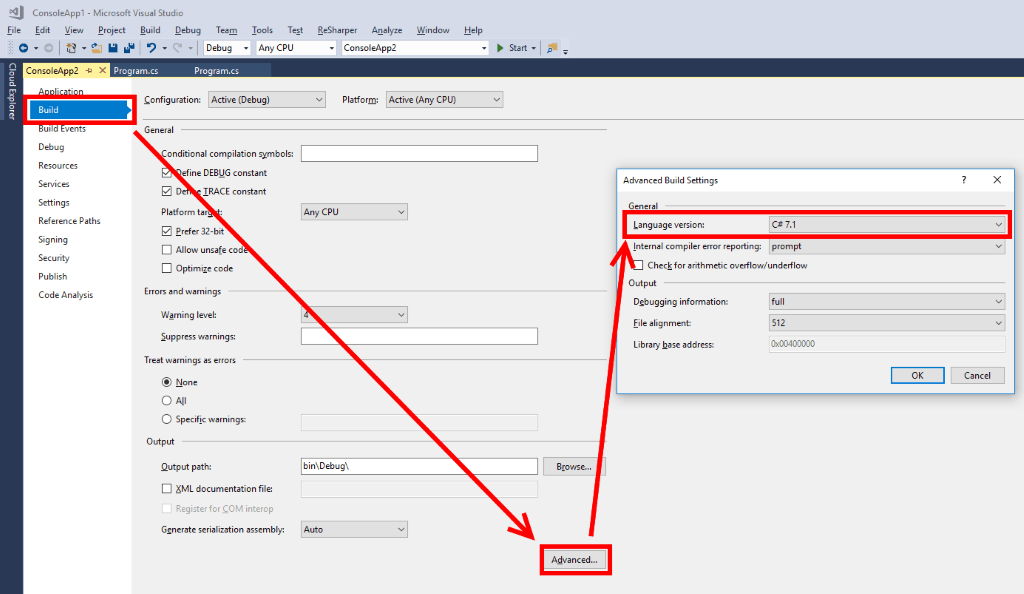
Now the new features of C# 7.1 including asynchronous main methods are available.
C# 7.1 Async Main Methods
With C# 7.0, in a console app the entry point could not be marked async requiring some workarounds/boilerplate code, for example:
class Program
{
static void Main(string[] args)
{
MainAsync().GetAwaiter().GetResult();
}
private static async Task MainAsync()
{
using (StreamWriter writer = File.CreateText(@"c:\temp\anewfile.txt"))
{
await writer.WriteLineAsync("Hello");
}
}
}
With C# 7.1, the main method can be async, as the following code shows:
class Program
{
static async Task Main(string[] args)
{
using (StreamWriter writer = File.CreateText(@"c:\temp\anewfile.txt"))
{
await writer.WriteLineAsync("Hello");
}
}
}
You can check out the C#7.1 features on GitHub such as:
If you want to fill in the gaps in your C# knowledge be sure to check out my C# Tips and Traps training course from Pluralsight – get started with a free trial.


SHARE: