Using the Console.SetIn Method allows us to specify an alternative source (TextReader stream).
For example suppose we have the following “names.txt” text file:
Sarah
Amrit
Gentry
Jack
We can create a new stream that reads from this file whenever we perform a Console.ReadLine(). Now when we call ReadLine, a line is read from the text file rather than the keyboard.
We can use the Console.OpenStandardInput() method to reset input back to the keyboard.
The code below creates the following output:
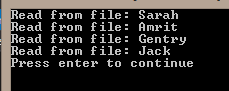
class Program
{
static void Main(string[] args)
{
// IO exceptions/etc. checking code omitted
var inputNames = new StreamReader(@"c:\temp\names.txt");
// Tell console to get its input from the file, not from the keyboard
Console.SetIn(inputNames);
// Output each line of the file until no more lines
string currentName = Console.ReadLine();
while (currentName != null)
{
Console.WriteLine("Read from file: " + currentName);
currentName = Console.ReadLine();
}
Console.WriteLine("Press enter to continue");
// Reset console input to the standard (keyboard) input so we can wait for keyboard enter
Console.SetIn(new StreamReader(Console.OpenStandardInput()));
// Wait for keyboard enter
Console.ReadLine();
}
}
For more console related tips, check out my Building .NET Console Applications in C# Pluralsight course.
You can start watching with a Pluralsight free trial.


SHARE: