LinqToTwitter is an open source library to help work with the Twitter API. It can be used in a Windows Store app to authorise a user and your app and allow querying and sending of Tweets.
Setting Up
First install the NuGet package into your Windows Store app. You’ll then need to head over to https://dev.twitter.com/ and sign in and define an app – you’ll end up with a couple of items that you’ll need later to authorise your app to use the API: API Key (aka consumer key) & API Secret (aka consumer secret).
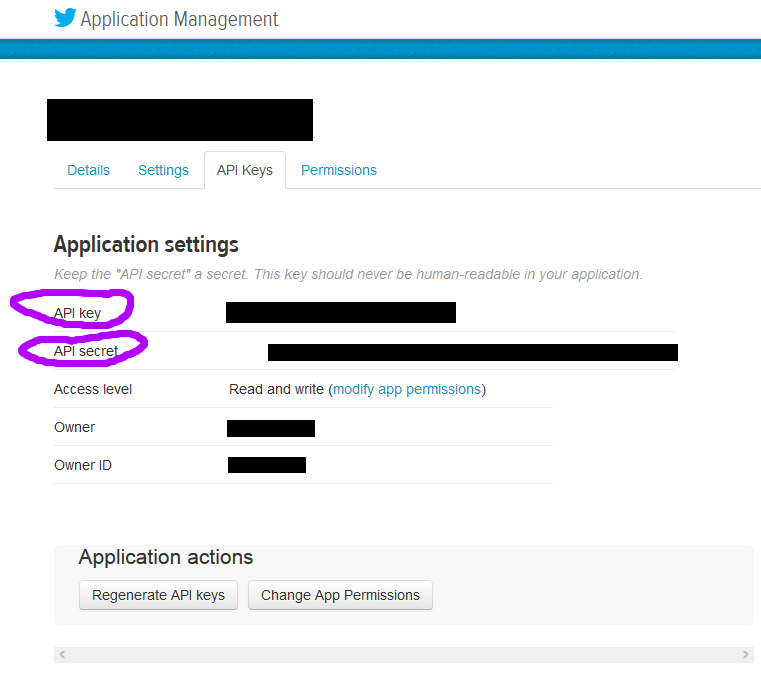
Authorising Your App for a Given User
The first step is that the user must sign in to Twitter and grant your app access to their Twitter account.
First include the namespaces: LinqToTwitter and LinqToTwitter.WindowsStore.
Next create an instance of an InMemoryCredentialStore and set the consumer key and secret for your app as defined above.
var c = new InMemoryCredentialStore
{
ConsumerKey = "your API key here",
ConsumerSecret = "your API secret here"
};
Next, create a WindowsStoreAuthorizer and use the InMemoryCredentialStore and a callback address – this callback doesn’t actually have to do anything):
var authorizer = new WindowsStoreAuthorizer
{
CredentialStore = c,
Callback = "http://somecallbackaddresshere.com/blah.html"
};
Now calling the AuthorizeAsync method will bring up the Twitter auth dialog.
await authorizer.AuthorizeAsync();
Following the await, if the auth succeeded you can get the OAuthToken and OAuthTokenSecret with: authorizer.CredentialStore.OAuthToken and authorizer.CredentialStore.OAuthTokenSecret.
Sending a Tweet
To send a Tweet, an instance of a TwitterContext is needed. To create this an authorizer is passed to its constructor. You can use the instance (“authorizer”) you already have, or if you’re sending from a different part of the app codebase you may need to re-create one. To do this:
var authorizer = new WindowsStoreAuthorizer
{
CredentialStore = new InMemoryCredentialStore
{
ConsumerKey = "your key",
ConsumerSecret = "your secret",
OAuthToken = token received from AuthorizeAsync,
OAuthTokenSecret = token received from AuthorizeAsync
}
};
To create the context:
var context = new TwitterContext(authorizer);
Now that we have a context, we can use it to send a new Tweet:
await _context.TweetAsync("tweet content here");
SHARE: