Visual Studio can be used to create precompiled Azure Functions using standard C# classes and tools/techniques and then they can be published to Azure.
This article assumes you’ve created the resources (resource group, Event Grid Topic, etc.) from this previous article.
In Visual Studio 2017, create a new Azure Functions project.
Next update the pre-installed Microsoft.NET.Sdk.Functions NuGet package to the latest version.
To get access to the Azure Event Grid function trigger attribute, install the Microsoft.Azure.WebJobs.Extensions.EventGrid NuGet package (this package is currently in preview/beta).
Add a new class to the project with the following code:
using Microsoft.Azure.WebJobs;
using Microsoft.Azure.WebJobs.Extensions.EventGrid;
using Microsoft.Azure.WebJobs.Host;
namespace DCTDemos
{
public static class Class1
{
[FunctionName("SendNewLeadWelcomeLetter")]
public static void SendNewLeadWelcomeLetter([EventGridTrigger] EventGridEvent eventGridEvent, TraceWriter log)
{
log.Info($"EventGridEvent" +
$"\n\tId:{eventGridEvent.Id}" +
$"\n\tTopic:{eventGridEvent.Topic}" +
$"\n\tSubject:{eventGridEvent.Subject}" +
$"\n\tType:{eventGridEvent.EventType}" +
$"\n\tData:{eventGridEvent.Data}");
}
}
}
Notice in the preceding code, the method name SendNewLeadWelcomeLetter is the same as specified in the function name attribute, this may be required due to a bug in the current preview/beta implementation – if these are different your function may not be executed when an event occurs.
Right-click on the function project and choose publish. Follow the wizard and create a new Function App and select your resource group where your Event Grid Topics is. Select West US 2 if you need to create any new Azure resources/storage account/etc..
Once deployed, head over to Azure Portal, open your new function app and select the newly deployed SendNewLeadWelcomeLetter function:
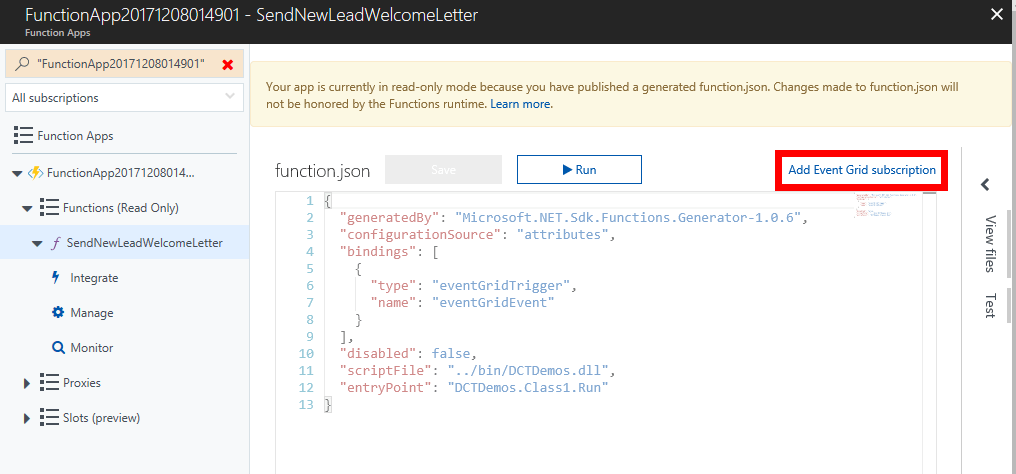
At the top right select Add Event Grid subscription. And follow the wizard to create a new subscription - this will enable the new function to be triggered by an Event Grid Subscription. As part of the subscription we’ll limit the event type to new-sales-lead-created:
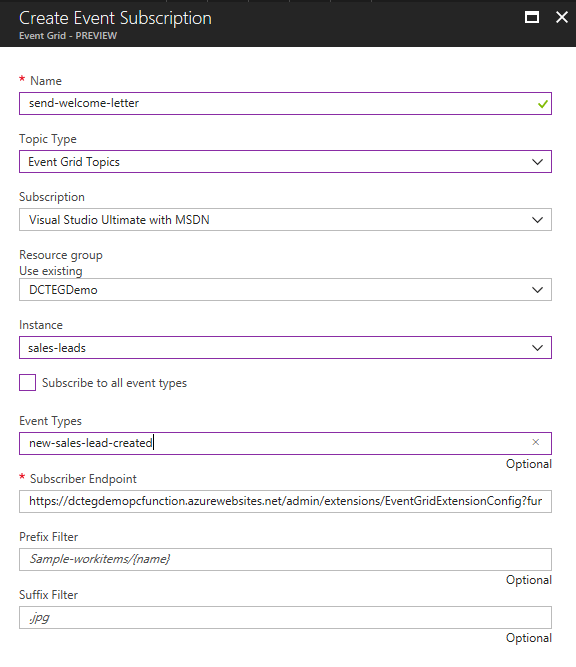
Next go to the function app platform features tab and select Log Streaming. We can now use Postman to POST the following JSON to the Event Grid Topic we created earlier.
[
{
"id": "1236",
"eventType": "new-sales-lead-created",
"subject": "myapp/sales/leads",
"eventTime": "2017-12-08T01:01:36+00:00",
"data":{
"firstName": "Amrit",
"postalAddress": "xyz"
}
}
]
Head back to the streaming logs and you should see your precompiled Azure Function executing in response to the Event Grid event:
2017-12-08T06:38:25 Welcome, you are now connected to log-streaming service.
2017-12-08T06:38:49.841 Function started (Id=ec927bc1-fa15-4211-a7bd-8e593f5d4840)
2017-12-08T06:38:49.841 EventGridEvent
Id:1234
Topic:/subscriptions/797e1c4e-3fd4-4cd6-84b8-ef103cee8b6b/resourceGroups/DCTEGDemo/providers/Microsoft.EventGrid/topics/sales-leads
Subject:myapp/sales/leads
Type:new-sales-lead-created
Data:{
"firstName": "Amrit",
"postalAddress": "xyz"
}
2017-12-08T06:38:49.841 Function completed (Success, Id=ec927bc1-fa15-4211-a7bd-8e593f5d4840, Duration=0ms)
To learn how to create precompiled Azure Functions in Visual Studio, check out my Writing and Testing Precompiled Azure Functions in Visual Studio 2017 Pluralsight course.
You can start watching with a Pluralsight free trial.


SHARE: