I though it would be interesting to see how easy it is to define an MVVM Light view model once and then use it in both a Universal Windows Phone and Windows Store project.
So I created a new blank universal apps project and added the “MVVM Light libraries only (PCL) NuGet” package to both the Windows 8.1 project and the Windows Phone 8.1 project.
Next create a new view model class in the shared project:
using System.Linq;
using GalaSoft.MvvmLight;
using GalaSoft.MvvmLight.Command;
namespace UniMvvmLight
{
class MainViewModel : ViewModelBase
{
public MainViewModel()
{
Words = "Hello";
Reverse = new RelayCommand(() =>
{
Words = new string(Words.ToCharArray().Reverse().ToArray());
});
}
private string _words;
public RelayCommand Reverse { get; set; }
public string Words
{
get
{
return _words;
}
set
{
Set(() => Words, ref _words, value);
}
}
}
}
I’m not going to create a view model locator etc, just simply set the datacontext in the code behind of both the phone and store MainWindow:
public MainPage()
{
this.InitializeComponent();
this.DataContext = new MainViewModel();
}
Next define the xaml in both the MainWindow.xaml in both phone and store projects:
<Grid>
<StackPanel>
<TextBox Text="{Binding Words}"></TextBox>
<Button Command="{Binding Reverse}">Reverse</Button>
</StackPanel>
</Grid>
Now running either the phone or store app works as expected, the view model binding works as expected and clicking the button reverses the string as expected. Whilst this is not surprising, the view model in the shared project is just like having a copy of it in both app projects, even so it’s still cool.
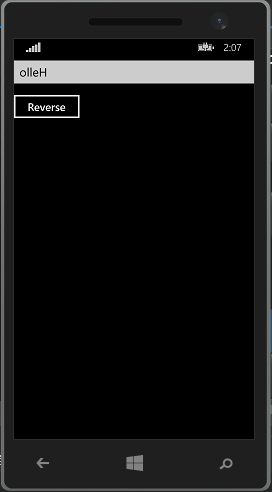
If we wanted to we could then leverage C# partial classes and methods to define platform-specific viewmodel code.
SHARE: